- User Guide
- Indexing...
Indexing and selecting data#
The axis labeling information in pandas objects serves many purposes:
Identifies data (i.e. providesmetadata) using known indicators,important for analysis, visualization, and interactive console display.
Enables automatic and explicit data alignment.
Allows intuitive getting and setting of subsets of the data set.
In this section, we will focus on the final point: namely, how to slice, dice,and generally get and set subsets of pandas objects. The primary focus will beon Series and DataFrame as they have received more development attention inthis area.
Note
The Python and NumPy indexing operators[]
and attribute operator.
provide quick and easy access to pandas data structures across a wide rangeof use cases. This makes interactive work intuitive, as there’s little newto learn if you already know how to deal with Python dictionaries and NumPyarrays. However, since the type of the data to be accessed isn’t known inadvance, directly using standard operators has some optimization limits. Forproduction code, we recommended that you take advantage of the optimizedpandas data access methods exposed in this chapter.
Warning
Whether a copy or a reference is returned for a setting operation, maydepend on the context. This is sometimes calledchained assignment andshould be avoided. SeeReturning a View versus Copy.
See theMultiIndex / Advanced Indexing forMultiIndex
and more advanced indexing documentation.
See thecookbook for some advanced strategies.
Different choices for indexing#
Object selection has had a number of user-requested additions in order tosupport more explicit location based indexing. pandas now supports three typesof multi-axis indexing.
.loc
is primarily label based, but may also be used with a boolean array..loc
will raiseKeyError
when the items are not found. Allowed inputs are:A single label, e.g.
5
or'a'
(Note that5
is interpreted as alabel of the index. This use isnot an integer position along theindex.).A list or array of labels
['a','b','c']
.A slice object with labels
'a':'f'
(Note that contrary to usual Pythonslices,both the start and the stop are included, when present in theindex! SeeSlicing with labelsandEndpoints are inclusive.)A boolean array (any
NA
values will be treated asFalse
).A
callable
function with one argument (the calling Series or DataFrame) andthat returns valid output for indexing (one of the above).A tuple of row (and column) indices whose elements are one of theabove inputs.
See more atSelection by Label.
.iloc
is primarily integer position based (from0
tolength-1
of the axis), but may also be used with a booleanarray..iloc
will raiseIndexError
if a requestedindexer is out-of-bounds, exceptslice indexers which allowout-of-bounds indexing. (this conforms with Python/NumPyslicesemantics). Allowed inputs are:An integer e.g.
5
.A list or array of integers
[4,3,0]
.A slice object with ints
1:7
.A boolean array (any
NA
values will be treated asFalse
).A
callable
function with one argument (the calling Series or DataFrame) andthat returns valid output for indexing (one of the above).A tuple of row (and column) indices whose elements are one of theabove inputs.
See more atSelection by Position,Advanced Indexing andAdvancedHierarchical.
.loc
,.iloc
, and also[]
indexing can accept acallable
as indexer. See more atSelection By Callable.Note
Destructuring tuple keys into row (and column) indexes occursbefore callables are applied, so you cannot return a tuple froma callable to index both rows and columns.
Getting values from an object with multi-axes selection uses the followingnotation (using.loc
as an example, but the following applies to.iloc
aswell). Any of the axes accessors may be the null slice:
. Axes left out ofthe specification are assumed to be:
, e.g.p.loc['a']
is equivalent top.loc['a',:]
.
In [1]:ser=pd.Series(range(5),index=list("abcde"))In [2]:ser.loc[["a","c","e"]]Out[2]:a 0c 2e 4dtype: int64In [3]:df=pd.DataFrame(np.arange(25).reshape(5,5),index=list("abcde"),columns=list("abcde"))In [4]:df.loc[["a","c","e"],["b","d"]]Out[4]: b da 1 3c 11 13e 21 23
Basics#
As mentioned when introducing the data structures in thelast section, the primary function of indexing with[]
(a.k.a.__getitem__
for those familiar with implementing class behavior in Python) is selecting outlower-dimensional slices. The following table shows return type values whenindexing pandas objects with[]
:
Object Type | Selection | Return Value Type |
---|---|---|
Series |
| scalar value |
DataFrame |
|
|
Here we construct a simple time series data set to use for illustrating theindexing functionality:
In [5]:dates=pd.date_range('1/1/2000',periods=8)In [6]:df=pd.DataFrame(np.random.randn(8,4), ...:index=dates,columns=['A','B','C','D']) ...:In [7]:dfOut[7]: A B C D2000-01-01 0.469112 -0.282863 -1.509059 -1.1356322000-01-02 1.212112 -0.173215 0.119209 -1.0442362000-01-03 -0.861849 -2.104569 -0.494929 1.0718042000-01-04 0.721555 -0.706771 -1.039575 0.2718602000-01-05 -0.424972 0.567020 0.276232 -1.0874012000-01-06 -0.673690 0.113648 -1.478427 0.5249882000-01-07 0.404705 0.577046 -1.715002 -1.0392682000-01-08 -0.370647 -1.157892 -1.344312 0.844885
Note
None of the indexing functionality is time series specific unlessspecifically stated.
Thus, as per above, we have the most basic indexing using[]
:
In [8]:s=df['A']In [9]:s[dates[5]]Out[9]:-0.6736897080883706
You can pass a list of columns to[]
to select columns in that order.If a column is not contained in the DataFrame, an exception will beraised. Multiple columns can also be set in this manner:
In [10]:dfOut[10]: A B C D2000-01-01 0.469112 -0.282863 -1.509059 -1.1356322000-01-02 1.212112 -0.173215 0.119209 -1.0442362000-01-03 -0.861849 -2.104569 -0.494929 1.0718042000-01-04 0.721555 -0.706771 -1.039575 0.2718602000-01-05 -0.424972 0.567020 0.276232 -1.0874012000-01-06 -0.673690 0.113648 -1.478427 0.5249882000-01-07 0.404705 0.577046 -1.715002 -1.0392682000-01-08 -0.370647 -1.157892 -1.344312 0.844885In [11]:df[['B','A']]=df[['A','B']]In [12]:dfOut[12]: A B C D2000-01-01 -0.282863 0.469112 -1.509059 -1.1356322000-01-02 -0.173215 1.212112 0.119209 -1.0442362000-01-03 -2.104569 -0.861849 -0.494929 1.0718042000-01-04 -0.706771 0.721555 -1.039575 0.2718602000-01-05 0.567020 -0.424972 0.276232 -1.0874012000-01-06 0.113648 -0.673690 -1.478427 0.5249882000-01-07 0.577046 0.404705 -1.715002 -1.0392682000-01-08 -1.157892 -0.370647 -1.344312 0.844885
You may find this useful for applying a transform (in-place) to a subset of thecolumns.
Warning
pandas aligns all AXES when settingSeries
andDataFrame
from.loc
.
This willnot modifydf
because the column alignment is before value assignment.
In [13]:df[['A','B']]Out[13]: A B2000-01-01 -0.282863 0.4691122000-01-02 -0.173215 1.2121122000-01-03 -2.104569 -0.8618492000-01-04 -0.706771 0.7215552000-01-05 0.567020 -0.4249722000-01-06 0.113648 -0.6736902000-01-07 0.577046 0.4047052000-01-08 -1.157892 -0.370647In [14]:df.loc[:,['B','A']]=df[['A','B']]In [15]:df[['A','B']]Out[15]: A B2000-01-01 -0.282863 0.4691122000-01-02 -0.173215 1.2121122000-01-03 -2.104569 -0.8618492000-01-04 -0.706771 0.7215552000-01-05 0.567020 -0.4249722000-01-06 0.113648 -0.6736902000-01-07 0.577046 0.4047052000-01-08 -1.157892 -0.370647
The correct way to swap column values is by using raw values:
In [16]:df.loc[:,['B','A']]=df[['A','B']].to_numpy()In [17]:df[['A','B']]Out[17]: A B2000-01-01 0.469112 -0.2828632000-01-02 1.212112 -0.1732152000-01-03 -0.861849 -2.1045692000-01-04 0.721555 -0.7067712000-01-05 -0.424972 0.5670202000-01-06 -0.673690 0.1136482000-01-07 0.404705 0.5770462000-01-08 -0.370647 -1.157892
However, pandas does not align AXES when settingSeries
andDataFrame
from.iloc
because.iloc
operates by position.
This will modifydf
because the column alignment is not done before value assignment.
In [18]:df[['A','B']]Out[18]: A B2000-01-01 0.469112 -0.2828632000-01-02 1.212112 -0.1732152000-01-03 -0.861849 -2.1045692000-01-04 0.721555 -0.7067712000-01-05 -0.424972 0.5670202000-01-06 -0.673690 0.1136482000-01-07 0.404705 0.5770462000-01-08 -0.370647 -1.157892In [19]:df.iloc[:,[1,0]]=df[['A','B']]In [20]:df[['A','B']]Out[20]: A B2000-01-01 -0.282863 0.4691122000-01-02 -0.173215 1.2121122000-01-03 -2.104569 -0.8618492000-01-04 -0.706771 0.7215552000-01-05 0.567020 -0.4249722000-01-06 0.113648 -0.6736902000-01-07 0.577046 0.4047052000-01-08 -1.157892 -0.370647
Attribute access#
You may access an index on aSeries
or column on aDataFrame
directlyas an attribute:
In [21]:sa=pd.Series([1,2,3],index=list('abc'))In [22]:dfa=df.copy()
In [23]:sa.bOut[23]:2In [24]:dfa.AOut[24]:2000-01-01 -0.2828632000-01-02 -0.1732152000-01-03 -2.1045692000-01-04 -0.7067712000-01-05 0.5670202000-01-06 0.1136482000-01-07 0.5770462000-01-08 -1.157892Freq: D, Name: A, dtype: float64
In [25]:sa.a=5In [26]:saOut[26]:a 5b 2c 3dtype: int64In [27]:dfa.A=list(range(len(dfa.index)))# ok if A already existsIn [28]:dfaOut[28]: A B C D2000-01-01 0 0.469112 -1.509059 -1.1356322000-01-02 1 1.212112 0.119209 -1.0442362000-01-03 2 -0.861849 -0.494929 1.0718042000-01-04 3 0.721555 -1.039575 0.2718602000-01-05 4 -0.424972 0.276232 -1.0874012000-01-06 5 -0.673690 -1.478427 0.5249882000-01-07 6 0.404705 -1.715002 -1.0392682000-01-08 7 -0.370647 -1.344312 0.844885In [29]:dfa['A']=list(range(len(dfa.index)))# use this form to create a new columnIn [30]:dfaOut[30]: A B C D2000-01-01 0 0.469112 -1.509059 -1.1356322000-01-02 1 1.212112 0.119209 -1.0442362000-01-03 2 -0.861849 -0.494929 1.0718042000-01-04 3 0.721555 -1.039575 0.2718602000-01-05 4 -0.424972 0.276232 -1.0874012000-01-06 5 -0.673690 -1.478427 0.5249882000-01-07 6 0.404705 -1.715002 -1.0392682000-01-08 7 -0.370647 -1.344312 0.844885
Warning
You can use this access only if the index element is a valid Python identifier, e.g.
s.1
is not allowed.Seehere for an explanation of valid identifiers.The attribute will not be available if it conflicts with an existing method name, e.g.
s.min
is not allowed, buts['min']
is possible.Similarly, the attribute will not be available if it conflicts with any of the following list:
index
,major_axis
,minor_axis
,items
.In any of these cases, standard indexing will still work, e.g.
s['1']
,s['min']
, ands['index']
willaccess the corresponding element or column.
If you are using the IPython environment, you may also use tab-completion tosee these accessible attributes.
You can also assign adict
to a row of aDataFrame
:
In [31]:x=pd.DataFrame({'x':[1,2,3],'y':[3,4,5]})In [32]:x.iloc[1]={'x':9,'y':99}In [33]:xOut[33]: x y0 1 31 9 992 3 5
You can use attribute access to modify an existing element of a Series or column of a DataFrame, but be careful;if you try to use attribute access to create a new column, it creates a new attribute rather than anew column and will this raise aUserWarning
:
In [34]:df_new=pd.DataFrame({'one':[1.,2.,3.]})In [35]:df_new.two=[4,5,6]In [36]:df_newOut[36]: one0 1.01 2.02 3.0
Slicing ranges#
The most robust and consistent way of slicing ranges along arbitrary axes isdescribed in theSelection by Position sectiondetailing the.iloc
method. For now, we explain the semantics of slicing using the[]
operator.
With Series, the syntax works exactly as with an ndarray, returning a slice ofthe values and the corresponding labels:
In [37]:s[:5]Out[37]:2000-01-01 0.4691122000-01-02 1.2121122000-01-03 -0.8618492000-01-04 0.7215552000-01-05 -0.424972Freq: D, Name: A, dtype: float64In [38]:s[::2]Out[38]:2000-01-01 0.4691122000-01-03 -0.8618492000-01-05 -0.4249722000-01-07 0.404705Freq: 2D, Name: A, dtype: float64In [39]:s[::-1]Out[39]:2000-01-08 -0.3706472000-01-07 0.4047052000-01-06 -0.6736902000-01-05 -0.4249722000-01-04 0.7215552000-01-03 -0.8618492000-01-02 1.2121122000-01-01 0.469112Freq: -1D, Name: A, dtype: float64
Note that setting works as well:
In [40]:s2=s.copy()In [41]:s2[:5]=0In [42]:s2Out[42]:2000-01-01 0.0000002000-01-02 0.0000002000-01-03 0.0000002000-01-04 0.0000002000-01-05 0.0000002000-01-06 -0.6736902000-01-07 0.4047052000-01-08 -0.370647Freq: D, Name: A, dtype: float64
With DataFrame, slicing inside of[]
slices the rows. This is providedlargely as a convenience since it is such a common operation.
In [43]:df[:3]Out[43]: A B C D2000-01-01 -0.282863 0.469112 -1.509059 -1.1356322000-01-02 -0.173215 1.212112 0.119209 -1.0442362000-01-03 -2.104569 -0.861849 -0.494929 1.071804In [44]:df[::-1]Out[44]: A B C D2000-01-08 -1.157892 -0.370647 -1.344312 0.8448852000-01-07 0.577046 0.404705 -1.715002 -1.0392682000-01-06 0.113648 -0.673690 -1.478427 0.5249882000-01-05 0.567020 -0.424972 0.276232 -1.0874012000-01-04 -0.706771 0.721555 -1.039575 0.2718602000-01-03 -2.104569 -0.861849 -0.494929 1.0718042000-01-02 -0.173215 1.212112 0.119209 -1.0442362000-01-01 -0.282863 0.469112 -1.509059 -1.135632
Selection by label#
Warning
Whether a copy or a reference is returned for a setting operation, may depend on the context.This is sometimes calledchainedassignment
and should be avoided.SeeReturning a View versus Copy.
Warning
.loc
is strict when you present slicers that are not compatible (or convertible) with the index type. For exampleusing integers in aDatetimeIndex
. These will raise aTypeError
.In [45]:dfl=pd.DataFrame(np.random.randn(5,4), ....:columns=list('ABCD'), ....:index=pd.date_range('20130101',periods=5)) ....:In [46]:dflOut[46]: A B C D2013-01-01 1.075770 -0.109050 1.643563 -1.4693882013-01-02 0.357021 -0.674600 -1.776904 -0.9689142013-01-03 -1.294524 0.413738 0.276662 -0.4720352013-01-04 -0.013960 -0.362543 -0.006154 -0.9230612013-01-05 0.895717 0.805244 -1.206412 2.565646In [47]:dfl.loc[2:3]---------------------------------------------------------------------------TypeErrorTraceback (most recent call last)CellIn[47],line1---->1dfl.loc[2:3]File ~/work/pandas/pandas/pandas/core/indexing.py:1191, in_LocationIndexer.__getitem__(self, key)1189maybe_callable=com.apply_if_callable(key,self.obj)1190maybe_callable=self._check_deprecated_callable_usage(key,maybe_callable)->1191returnself._getitem_axis(maybe_callable,axis=axis)File ~/work/pandas/pandas/pandas/core/indexing.py:1411, in_LocIndexer._getitem_axis(self, key, axis)1409ifisinstance(key,slice):1410self._validate_key(key,axis)->1411returnself._get_slice_axis(key,axis=axis)1412elifcom.is_bool_indexer(key):1413returnself._getbool_axis(key,axis=axis)File ~/work/pandas/pandas/pandas/core/indexing.py:1443, in_LocIndexer._get_slice_axis(self, slice_obj, axis)1440returnobj.copy(deep=False)1442labels=obj._get_axis(axis)->1443indexer=labels.slice_indexer(slice_obj.start,slice_obj.stop,slice_obj.step)1445ifisinstance(indexer,slice):1446returnself.obj._slice(indexer,axis=axis)File ~/work/pandas/pandas/pandas/core/indexes/datetimes.py:682, inDatetimeIndex.slice_indexer(self, start, end, step)674# GH#33146 if start and end are combinations of str and None and Index is not675# monotonic, we can not use Index.slice_indexer because it does not honor the676# actual elements, is only searching for start and end677if(678check_str_or_none(start)679orcheck_str_or_none(end)680orself.is_monotonic_increasing681):-->682returnIndex.slice_indexer(self,start,end,step)684mask=np.array(True)685in_index=TrueFile ~/work/pandas/pandas/pandas/core/indexes/base.py:6708, inIndex.slice_indexer(self, start, end, step)6664defslice_indexer(6665self,6666start:Hashable|None=None,6667end:Hashable|None=None,6668step:int|None=None,6669)->slice:6670"""6671 Compute the slice indexer for input labels and step.6672 (...)6706 slice(1, 3, None)6707 """->6708start_slice,end_slice=self.slice_locs(start,end,step=step)6710# return a slice6711ifnotis_scalar(start_slice):File ~/work/pandas/pandas/pandas/core/indexes/base.py:6934, inIndex.slice_locs(self, start, end, step)6932start_slice=None6933ifstartisnotNone:->6934start_slice=self.get_slice_bound(start,"left")6935ifstart_sliceisNone:6936start_slice=0File ~/work/pandas/pandas/pandas/core/indexes/base.py:6849, inIndex.get_slice_bound(self, label, side)6845original_label=label6847# For datetime indices label may be a string that has to be converted6848# to datetime boundary according to its resolution.->6849label=self._maybe_cast_slice_bound(label,side)6851# we need to look up the label6852try:File ~/work/pandas/pandas/pandas/core/indexes/datetimes.py:642, inDatetimeIndex._maybe_cast_slice_bound(self, label, side)637ifisinstance(label,dt.date)andnotisinstance(label,dt.datetime):638# Pandas supports slicing with dates, treated as datetimes at midnight.639# https://github.com/pandas-dev/pandas/issues/31501640label=Timestamp(label).to_pydatetime()-->642label=super()._maybe_cast_slice_bound(label,side)643self._data._assert_tzawareness_compat(label)644returnTimestamp(label)File ~/work/pandas/pandas/pandas/core/indexes/datetimelike.py:378, inDatetimeIndexOpsMixin._maybe_cast_slice_bound(self, label, side)376returnlowerifside=="left"elseupper377elifnotisinstance(label,self._data._recognized_scalars):-->378self._raise_invalid_indexer("slice",label)380returnlabelFile ~/work/pandas/pandas/pandas/core/indexes/base.py:4308, inIndex._raise_invalid_indexer(self, form, key, reraise)4306ifreraiseisnotlib.no_default:4307raiseTypeError(msg)fromreraise->4308raiseTypeError(msg)TypeError: cannot do slice indexing on DatetimeIndex with these indexers [2] of type int
String likes in slicingcan be convertible to the type of the index and lead to natural slicing.
In [48]:dfl.loc['20130102':'20130104']Out[48]: A B C D2013-01-02 0.357021 -0.674600 -1.776904 -0.9689142013-01-03 -1.294524 0.413738 0.276662 -0.4720352013-01-04 -0.013960 -0.362543 -0.006154 -0.923061
pandas provides a suite of methods in order to havepurely label based indexing. This is a strict inclusion based protocol.Every label asked for must be in the index, or aKeyError
will be raised.When slicing, both the start boundAND the stop bound areincluded, if present in the index.Integers are valid labels, but they refer to the labeland not the position.
The.loc
attribute is the primary access method. The following are valid inputs:
A single label, e.g.
5
or'a'
(Note that5
is interpreted as alabel of the index. This use isnot an integer position along the index.).A list or array of labels
['a','b','c']
.A slice object with labels
'a':'f'
(Note that contrary to usual Pythonslices,both the start and the stop are included, when present in theindex! SeeSlicing with labels.A boolean array.
A
callable
, seeSelection By Callable.
In [49]:s1=pd.Series(np.random.randn(6),index=list('abcdef'))In [50]:s1Out[50]:a 1.431256b 1.340309c -1.170299d -0.226169e 0.410835f 0.813850dtype: float64In [51]:s1.loc['c':]Out[51]:c -1.170299d -0.226169e 0.410835f 0.813850dtype: float64In [52]:s1.loc['b']Out[52]:1.3403088497993827
Note that setting works as well:
In [53]:s1.loc['c':]=0In [54]:s1Out[54]:a 1.431256b 1.340309c 0.000000d 0.000000e 0.000000f 0.000000dtype: float64
With a DataFrame:
In [55]:df1=pd.DataFrame(np.random.randn(6,4), ....:index=list('abcdef'), ....:columns=list('ABCD')) ....:In [56]:df1Out[56]: A B C Da 0.132003 -0.827317 -0.076467 -1.187678b 1.130127 -1.436737 -1.413681 1.607920c 1.024180 0.569605 0.875906 -2.211372d 0.974466 -2.006747 -0.410001 -0.078638e 0.545952 -1.219217 -1.226825 0.769804f -1.281247 -0.727707 -0.121306 -0.097883In [57]:df1.loc[['a','b','d'],:]Out[57]: A B C Da 0.132003 -0.827317 -0.076467 -1.187678b 1.130127 -1.436737 -1.413681 1.607920d 0.974466 -2.006747 -0.410001 -0.078638
Accessing via label slices:
In [58]:df1.loc['d':,'A':'C']Out[58]: A B Cd 0.974466 -2.006747 -0.410001e 0.545952 -1.219217 -1.226825f -1.281247 -0.727707 -0.121306
For getting a cross section using a label (equivalent todf.xs('a')
):
In [59]:df1.loc['a']Out[59]:A 0.132003B -0.827317C -0.076467D -1.187678Name: a, dtype: float64
For getting values with a boolean array:
In [60]:df1.loc['a']>0Out[60]:A TrueB FalseC FalseD FalseName: a, dtype: boolIn [61]:df1.loc[:,df1.loc['a']>0]Out[61]: Aa 0.132003b 1.130127c 1.024180d 0.974466e 0.545952f -1.281247
NA values in a boolean array propagate asFalse
:
In [62]:mask=pd.array([True,False,True,False,pd.NA,False],dtype="boolean")In [63]:maskOut[63]:<BooleanArray>[True, False, True, False, <NA>, False]Length: 6, dtype: booleanIn [64]:df1[mask]Out[64]: A B C Da 0.132003 -0.827317 -0.076467 -1.187678c 1.024180 0.569605 0.875906 -2.211372
For getting a value explicitly:
# this is also equivalent to ``df1.at['a','A']``In [65]:df1.loc['a','A']Out[65]:0.13200317033032932
Slicing with labels#
When using.loc
with slices, if both the start and the stop labels arepresent in the index, then elementslocated between the two (including them)are returned:
In [66]:s=pd.Series(list('abcde'),index=[0,3,2,5,4])In [67]:s.loc[3:5]Out[67]:3 b2 c5 ddtype: object
If at least one of the two is absent, but the index is sorted, and can becompared against start and stop labels, then slicing will still work asexpected, by selecting labels whichrank between the two:
In [68]:s.sort_index()Out[68]:0 a2 c3 b4 e5 ddtype: objectIn [69]:s.sort_index().loc[1:6]Out[69]:2 c3 b4 e5 ddtype: object
However, if at least one of the two is absentand the index is not sorted, anerror will be raised (since doing otherwise would be computationally expensive,as well as potentially ambiguous for mixed type indexes). For instance, in theabove example,s.loc[1:6]
would raiseKeyError
.
For the rationale behind this behavior, seeEndpoints are inclusive.
In [70]:s=pd.Series(list('abcdef'),index=[0,3,2,5,4,2])In [71]:s.loc[3:5]Out[71]:3 b2 c5 ddtype: object
Also, if the index has duplicate labelsand either the start or the stop label is duplicated,an error will be raised. For instance, in the above example,s.loc[2:5]
would raise aKeyError
.
For more information about duplicate labels, seeDuplicate Labels.
Selection by position#
Warning
Whether a copy or a reference is returned for a setting operation, may depend on the context.This is sometimes calledchainedassignment
and should be avoided.SeeReturning a View versus Copy.
pandas provides a suite of methods in order to getpurely integer based indexing. The semantics follow closely Python and NumPy slicing. These are0-based
indexing. When slicing, the start bound isincluded, while the upper bound isexcluded. Trying to use a non-integer, even avalid label will raise anIndexError
.
The.iloc
attribute is the primary access method. The following are valid inputs:
An integer e.g.
5
.A list or array of integers
[4,3,0]
.A slice object with ints
1:7
.A boolean array.
A
callable
, seeSelection By Callable.A tuple of row (and column) indexes, whose elements are one of theabove types.
In [72]:s1=pd.Series(np.random.randn(5),index=list(range(0,10,2)))In [73]:s1Out[73]:0 0.6957752 0.3417344 0.9597266 -1.1103368 -0.619976dtype: float64In [74]:s1.iloc[:3]Out[74]:0 0.6957752 0.3417344 0.959726dtype: float64In [75]:s1.iloc[3]Out[75]:-1.110336102891167
Note that setting works as well:
In [76]:s1.iloc[:3]=0In [77]:s1Out[77]:0 0.0000002 0.0000004 0.0000006 -1.1103368 -0.619976dtype: float64
With a DataFrame:
In [78]:df1=pd.DataFrame(np.random.randn(6,4), ....:index=list(range(0,12,2)), ....:columns=list(range(0,8,2))) ....:In [79]:df1Out[79]: 0 2 4 60 0.149748 -0.732339 0.687738 0.1764442 0.403310 -0.154951 0.301624 -2.1798614 -1.369849 -0.954208 1.462696 -1.7431616 -0.826591 -0.345352 1.314232 0.6905798 0.995761 2.396780 0.014871 3.35742710 -0.317441 -1.236269 0.896171 -0.487602
Select via integer slicing:
In [80]:df1.iloc[:3]Out[80]: 0 2 4 60 0.149748 -0.732339 0.687738 0.1764442 0.403310 -0.154951 0.301624 -2.1798614 -1.369849 -0.954208 1.462696 -1.743161In [81]:df1.iloc[1:5,2:4]Out[81]: 4 62 0.301624 -2.1798614 1.462696 -1.7431616 1.314232 0.6905798 0.014871 3.357427
Select via integer list:
In [82]:df1.iloc[[1,3,5],[1,3]]Out[82]: 2 62 -0.154951 -2.1798616 -0.345352 0.69057910 -1.236269 -0.487602
In [83]:df1.iloc[1:3,:]Out[83]: 0 2 4 62 0.403310 -0.154951 0.301624 -2.1798614 -1.369849 -0.954208 1.462696 -1.743161
In [84]:df1.iloc[:,1:3]Out[84]: 2 40 -0.732339 0.6877382 -0.154951 0.3016244 -0.954208 1.4626966 -0.345352 1.3142328 2.396780 0.01487110 -1.236269 0.896171
# this is also equivalent to ``df1.iat[1,1]``In [85]:df1.iloc[1,1]Out[85]:-0.1549507744249032
For getting a cross section using an integer position (equiv todf.xs(1)
):
In [86]:df1.iloc[1]Out[86]:0 0.4033102 -0.1549514 0.3016246 -2.179861Name: 2, dtype: float64
Out of range slice indexes are handled gracefully just as in Python/NumPy.
# these are allowed in Python/NumPy.In [87]:x=list('abcdef')In [88]:xOut[88]:['a', 'b', 'c', 'd', 'e', 'f']In [89]:x[4:10]Out[89]:['e', 'f']In [90]:x[8:10]Out[90]:[]In [91]:s=pd.Series(x)In [92]:sOut[92]:0 a1 b2 c3 d4 e5 fdtype: objectIn [93]:s.iloc[4:10]Out[93]:4 e5 fdtype: objectIn [94]:s.iloc[8:10]Out[94]:Series([], dtype: object)
Note that using slices that go out of bounds can result inan empty axis (e.g. an empty DataFrame being returned).
In [95]:dfl=pd.DataFrame(np.random.randn(5,2),columns=list('AB'))In [96]:dflOut[96]: A B0 -0.082240 -2.1829371 0.380396 0.0848442 0.432390 1.5199703 -0.493662 0.6001784 0.274230 0.132885In [97]:dfl.iloc[:,2:3]Out[97]:Empty DataFrameColumns: []Index: [0, 1, 2, 3, 4]In [98]:dfl.iloc[:,1:3]Out[98]: B0 -2.1829371 0.0848442 1.5199703 0.6001784 0.132885In [99]:dfl.iloc[4:6]Out[99]: A B4 0.27423 0.132885
A single indexer that is out of bounds will raise anIndexError
.A list of indexers where any element is out of bounds will raise anIndexError
.
In [100]:dfl.iloc[[4,5,6]]---------------------------------------------------------------------------IndexErrorTraceback (most recent call last)File ~/work/pandas/pandas/pandas/core/indexing.py:1714, in_iLocIndexer._get_list_axis(self, key, axis)1713try:->1714returnself.obj._take_with_is_copy(key,axis=axis)1715exceptIndexErroraserr:1716# re-raise with different error message, e.g. test_getitem_ndarray_3dFile ~/work/pandas/pandas/pandas/core/generic.py:4172, inNDFrame._take_with_is_copy(self, indices, axis)4163"""4164 Internal version of the `take` method that sets the `_is_copy`4165 attribute to keep track of the parent dataframe (using in indexing (...)4170 See the docstring of `take` for full explanation of the parameters.4171 """->4172result=self.take(indices=indices,axis=axis)4173# Maybe set copy if we didn't actually change the index.File ~/work/pandas/pandas/pandas/core/generic.py:4152, inNDFrame.take(self, indices, axis, **kwargs)4148indices=np.arange(4149indices.start,indices.stop,indices.step,dtype=np.intp4150)->4152new_data=self._mgr.take(4153indices,4154axis=self._get_block_manager_axis(axis),4155verify=True,4156)4157returnself._constructor_from_mgr(new_data,axes=new_data.axes).__finalize__(4158self,method="take"4159)File ~/work/pandas/pandas/pandas/core/internals/managers.py:891, inBaseBlockManager.take(self, indexer, axis, verify)890n=self.shape[axis]-->891indexer=maybe_convert_indices(indexer,n,verify=verify)893new_labels=self.axes[axis].take(indexer)File ~/work/pandas/pandas/pandas/core/indexers/utils.py:282, inmaybe_convert_indices(indices, n, verify)281ifmask.any():-->282raiseIndexError("indices are out-of-bounds")283returnindicesIndexError: indices are out-of-boundsTheaboveexceptionwasthedirectcauseofthefollowingexception:IndexErrorTraceback (most recent call last)CellIn[100],line1---->1dfl.iloc[[4,5,6]]File ~/work/pandas/pandas/pandas/core/indexing.py:1191, in_LocationIndexer.__getitem__(self, key)1189maybe_callable=com.apply_if_callable(key,self.obj)1190maybe_callable=self._check_deprecated_callable_usage(key,maybe_callable)->1191returnself._getitem_axis(maybe_callable,axis=axis)File ~/work/pandas/pandas/pandas/core/indexing.py:1743, in_iLocIndexer._getitem_axis(self, key, axis)1741# a list of integers1742elifis_list_like_indexer(key):->1743returnself._get_list_axis(key,axis=axis)1745# a single integer1746else:1747key=item_from_zerodim(key)File ~/work/pandas/pandas/pandas/core/indexing.py:1717, in_iLocIndexer._get_list_axis(self, key, axis)1714returnself.obj._take_with_is_copy(key,axis=axis)1715exceptIndexErroraserr:1716# re-raise with different error message, e.g. test_getitem_ndarray_3d->1717raiseIndexError("positional indexers are out-of-bounds")fromerrIndexError: positional indexers are out-of-bounds
In [101]:dfl.iloc[:,4]---------------------------------------------------------------------------IndexErrorTraceback (most recent call last)CellIn[101],line1---->1dfl.iloc[:,4]File ~/work/pandas/pandas/pandas/core/indexing.py:1184, in_LocationIndexer.__getitem__(self, key)1182ifself._is_scalar_access(key):1183returnself.obj._get_value(*key,takeable=self._takeable)->1184returnself._getitem_tuple(key)1185else:1186# we by definition only have the 0th axis1187axis=self.axisor0File ~/work/pandas/pandas/pandas/core/indexing.py:1690, in_iLocIndexer._getitem_tuple(self, tup)1689def_getitem_tuple(self,tup:tuple):->1690tup=self._validate_tuple_indexer(tup)1691withsuppress(IndexingError):1692returnself._getitem_lowerdim(tup)File ~/work/pandas/pandas/pandas/core/indexing.py:966, in_LocationIndexer._validate_tuple_indexer(self, key)964fori,kinenumerate(key):965try:-->966self._validate_key(k,i)967exceptValueErroraserr:968raiseValueError(969"Location based indexing can only have "970f"[{self._valid_types}] types"971)fromerrFile ~/work/pandas/pandas/pandas/core/indexing.py:1592, in_iLocIndexer._validate_key(self, key, axis)1590return1591elifis_integer(key):->1592self._validate_integer(key,axis)1593elifisinstance(key,tuple):1594# a tuple should already have been caught by this point1595# so don't treat a tuple as a valid indexer1596raiseIndexingError("Too many indexers")File ~/work/pandas/pandas/pandas/core/indexing.py:1685, in_iLocIndexer._validate_integer(self, key, axis)1683len_axis=len(self.obj._get_axis(axis))1684ifkey>=len_axisorkey<-len_axis:->1685raiseIndexError("single positional indexer is out-of-bounds")IndexError: single positional indexer is out-of-bounds
Selection by callable#
.loc
,.iloc
, and also[]
indexing can accept acallable
as indexer.Thecallable
must be a function with one argument (the calling Series or DataFrame) that returns valid output for indexing.
Note
For.iloc
indexing, returning a tuple from the callable isnot supported, since tuple destructuring for row and column indexesoccursbefore applying callables.
In [102]:df1=pd.DataFrame(np.random.randn(6,4), .....:index=list('abcdef'), .....:columns=list('ABCD')) .....:In [103]:df1Out[103]: A B C Da -0.023688 2.410179 1.450520 0.206053b -0.251905 -2.213588 1.063327 1.266143c 0.299368 -0.863838 0.408204 -1.048089d -0.025747 -0.988387 0.094055 1.262731e 1.289997 0.082423 -0.055758 0.536580f -0.489682 0.369374 -0.034571 -2.484478In [104]:df1.loc[lambdadf:df['A']>0,:]Out[104]: A B C Dc 0.299368 -0.863838 0.408204 -1.048089e 1.289997 0.082423 -0.055758 0.536580In [105]:df1.loc[:,lambdadf:['A','B']]Out[105]: A Ba -0.023688 2.410179b -0.251905 -2.213588c 0.299368 -0.863838d -0.025747 -0.988387e 1.289997 0.082423f -0.489682 0.369374In [106]:df1.iloc[:,lambdadf:[0,1]]Out[106]: A Ba -0.023688 2.410179b -0.251905 -2.213588c 0.299368 -0.863838d -0.025747 -0.988387e 1.289997 0.082423f -0.489682 0.369374In [107]:df1[lambdadf:df.columns[0]]Out[107]:a -0.023688b -0.251905c 0.299368d -0.025747e 1.289997f -0.489682Name: A, dtype: float64
You can use callable indexing inSeries
.
In [108]:df1['A'].loc[lambdas:s>0]Out[108]:c 0.299368e 1.289997Name: A, dtype: float64
Using these methods / indexers, you can chain data selection operationswithout using a temporary variable.
In [109]:bb=pd.read_csv('data/baseball.csv',index_col='id')In [110]:(bb.groupby(['year','team']).sum(numeric_only=True) .....:.loc[lambdadf:df['r']>100]) .....:Out[110]: stint g ab r h X2b ... so ibb hbp sh sf gidpyear team ...2007 CIN 6 379 745 101 203 35 ... 127.0 14.0 1.0 1.0 15.0 18.0 DET 5 301 1062 162 283 54 ... 176.0 3.0 10.0 4.0 8.0 28.0 HOU 4 311 926 109 218 47 ... 212.0 3.0 9.0 16.0 6.0 17.0 LAN 11 413 1021 153 293 61 ... 141.0 8.0 9.0 3.0 8.0 29.0 NYN 13 622 1854 240 509 101 ... 310.0 24.0 23.0 18.0 15.0 48.0 SFN 5 482 1305 198 337 67 ... 188.0 51.0 8.0 16.0 6.0 41.0 TEX 2 198 729 115 200 40 ... 140.0 4.0 5.0 2.0 8.0 16.0 TOR 4 459 1408 187 378 96 ... 265.0 16.0 12.0 4.0 16.0 38.0[8 rows x 18 columns]
Combining positional and label-based indexing#
If you wish to get the 0th and the 2nd elements from the index in the ‘A’ column, you can do:
In [111]:dfd=pd.DataFrame({'A':[1,2,3], .....:'B':[4,5,6]}, .....:index=list('abc')) .....:In [112]:dfdOut[112]: A Ba 1 4b 2 5c 3 6In [113]:dfd.loc[dfd.index[[0,2]],'A']Out[113]:a 1c 3Name: A, dtype: int64
This can also be expressed using.iloc
, by explicitly getting locations on the indexers, and usingpositional indexing to select things.
In [114]:dfd.iloc[[0,2],dfd.columns.get_loc('A')]Out[114]:a 1c 3Name: A, dtype: int64
For gettingmultiple indexers, using.get_indexer
:
In [115]:dfd.iloc[[0,2],dfd.columns.get_indexer(['A','B'])]Out[115]: A Ba 1 4c 3 6
Reindexing#
The idiomatic way to achieve selecting potentially not-found elements is via.reindex()
. See also the section onreindexing.
In [116]:s=pd.Series([1,2,3])In [117]:s.reindex([1,2,3])Out[117]:1 2.02 3.03 NaNdtype: float64
Alternatively, if you want to select onlyvalid keys, the following is idiomatic and efficient; it is guaranteed to preserve the dtype of the selection.
In [118]:labels=[1,2,3]In [119]:s.loc[s.index.intersection(labels)]Out[119]:1 22 3dtype: int64
Having a duplicated index will raise for a.reindex()
:
In [120]:s=pd.Series(np.arange(4),index=['a','a','b','c'])In [121]:labels=['c','d']In [122]:s.reindex(labels)---------------------------------------------------------------------------ValueErrorTraceback (most recent call last)CellIn[122],line1---->1s.reindex(labels)File ~/work/pandas/pandas/pandas/core/series.py:5164, inSeries.reindex(self, index, axis, method, copy, level, fill_value, limit, tolerance)5147@doc(5148NDFrame.reindex,# type: ignore[has-type]5149klass=_shared_doc_kwargs["klass"],(...)5162tolerance=None,5163)->Series:->5164returnsuper().reindex(5165index=index,5166method=method,5167copy=copy,5168level=level,5169fill_value=fill_value,5170limit=limit,5171tolerance=tolerance,5172)File ~/work/pandas/pandas/pandas/core/generic.py:5629, inNDFrame.reindex(self, labels, index, columns, axis, method, copy, level, fill_value, limit, tolerance)5626returnself._reindex_multi(axes,copy,fill_value)5628# perform the reindex on the axes->5629returnself._reindex_axes(5630axes,level,limit,tolerance,method,fill_value,copy5631).__finalize__(self,method="reindex")File ~/work/pandas/pandas/pandas/core/generic.py:5652, inNDFrame._reindex_axes(self, axes, level, limit, tolerance, method, fill_value, copy)5649continue5651ax=self._get_axis(a)->5652new_index,indexer=ax.reindex(5653labels,level=level,limit=limit,tolerance=tolerance,method=method5654)5656axis=self._get_axis_number(a)5657obj=obj._reindex_with_indexers(5658{axis:[new_index,indexer]},5659fill_value=fill_value,5660copy=copy,5661allow_dups=False,5662)File ~/work/pandas/pandas/pandas/core/indexes/base.py:4436, inIndex.reindex(self, target, method, level, limit, tolerance)4433raiseValueError("cannot handle a non-unique multi-index!")4434elifnotself.is_unique:4435# GH#42568->4436raiseValueError("cannot reindex on an axis with duplicate labels")4437else:4438indexer,_=self.get_indexer_non_unique(target)ValueError: cannot reindex on an axis with duplicate labels
Generally, you can intersect the desired labels with the currentaxis, and then reindex.
In [123]:s.loc[s.index.intersection(labels)].reindex(labels)Out[123]:c 3.0d NaNdtype: float64
However, this wouldstill raise if your resulting index is duplicated.
In [124]:labels=['a','d']In [125]:s.loc[s.index.intersection(labels)].reindex(labels)---------------------------------------------------------------------------ValueErrorTraceback (most recent call last)CellIn[125],line1---->1s.loc[s.index.intersection(labels)].reindex(labels)File ~/work/pandas/pandas/pandas/core/series.py:5164, inSeries.reindex(self, index, axis, method, copy, level, fill_value, limit, tolerance)5147@doc(5148NDFrame.reindex,# type: ignore[has-type]5149klass=_shared_doc_kwargs["klass"],(...)5162tolerance=None,5163)->Series:->5164returnsuper().reindex(5165index=index,5166method=method,5167copy=copy,5168level=level,5169fill_value=fill_value,5170limit=limit,5171tolerance=tolerance,5172)File ~/work/pandas/pandas/pandas/core/generic.py:5629, inNDFrame.reindex(self, labels, index, columns, axis, method, copy, level, fill_value, limit, tolerance)5626returnself._reindex_multi(axes,copy,fill_value)5628# perform the reindex on the axes->5629returnself._reindex_axes(5630axes,level,limit,tolerance,method,fill_value,copy5631).__finalize__(self,method="reindex")File ~/work/pandas/pandas/pandas/core/generic.py:5652, inNDFrame._reindex_axes(self, axes, level, limit, tolerance, method, fill_value, copy)5649continue5651ax=self._get_axis(a)->5652new_index,indexer=ax.reindex(5653labels,level=level,limit=limit,tolerance=tolerance,method=method5654)5656axis=self._get_axis_number(a)5657obj=obj._reindex_with_indexers(5658{axis:[new_index,indexer]},5659fill_value=fill_value,5660copy=copy,5661allow_dups=False,5662)File ~/work/pandas/pandas/pandas/core/indexes/base.py:4436, inIndex.reindex(self, target, method, level, limit, tolerance)4433raiseValueError("cannot handle a non-unique multi-index!")4434elifnotself.is_unique:4435# GH#42568->4436raiseValueError("cannot reindex on an axis with duplicate labels")4437else:4438indexer,_=self.get_indexer_non_unique(target)ValueError: cannot reindex on an axis with duplicate labels
Selecting random samples#
A random selection of rows or columns from a Series or DataFrame with thesample()
method. The method will sample rows by default, and accepts a specific number of rows/columns to return, or a fraction of rows.
In [126]:s=pd.Series([0,1,2,3,4,5])# When no arguments are passed, returns 1 row.In [127]:s.sample()Out[127]:4 4dtype: int64# One may specify either a number of rows:In [128]:s.sample(n=3)Out[128]:0 04 41 1dtype: int64# Or a fraction of the rows:In [129]:s.sample(frac=0.5)Out[129]:5 53 31 1dtype: int64
By default,sample
will return each row at most once, but one can also sample with replacementusing thereplace
option:
In [130]:s=pd.Series([0,1,2,3,4,5])# Without replacement (default):In [131]:s.sample(n=6,replace=False)Out[131]:0 01 15 53 32 24 4dtype: int64# With replacement:In [132]:s.sample(n=6,replace=True)Out[132]:0 04 43 32 24 44 4dtype: int64
By default, each row has an equal probability of being selected, but if you want rowsto have different probabilities, you can pass thesample
function sampling weights asweights
. These weights can be a list, a NumPy array, or a Series, but they must be of the same length as the object you are sampling. Missing values will be treated as a weight of zero, and inf values are not allowed. If weights do not sum to 1, they will be re-normalized by dividing all weights by the sum of the weights. For example:
In [133]:s=pd.Series([0,1,2,3,4,5])In [134]:example_weights=[0,0,0.2,0.2,0.2,0.4]In [135]:s.sample(n=3,weights=example_weights)Out[135]:5 54 43 3dtype: int64# Weights will be re-normalized automaticallyIn [136]:example_weights2=[0.5,0,0,0,0,0]In [137]:s.sample(n=1,weights=example_weights2)Out[137]:0 0dtype: int64
When applied to a DataFrame, you can use a column of the DataFrame as sampling weights(provided you are sampling rows and not columns) by simply passing the name of the columnas a string.
In [138]:df2=pd.DataFrame({'col1':[9,8,7,6], .....:'weight_column':[0.5,0.4,0.1,0]}) .....:In [139]:df2.sample(n=3,weights='weight_column')Out[139]: col1 weight_column1 8 0.40 9 0.52 7 0.1
sample
also allows users to sample columns instead of rows using theaxis
argument.
In [140]:df3=pd.DataFrame({'col1':[1,2,3],'col2':[2,3,4]})In [141]:df3.sample(n=1,axis=1)Out[141]: col10 11 22 3
Finally, one can also set a seed forsample
’s random number generator using therandom_state
argument, which will accept either an integer (as a seed) or a NumPy RandomState object.
In [142]:df4=pd.DataFrame({'col1':[1,2,3],'col2':[2,3,4]})# With a given seed, the sample will always draw the same rows.In [143]:df4.sample(n=2,random_state=2)Out[143]: col1 col22 3 41 2 3In [144]:df4.sample(n=2,random_state=2)Out[144]: col1 col22 3 41 2 3
Setting with enlargement#
The.loc/[]
operations can perform enlargement when setting a non-existent key for that axis.
In theSeries
case this is effectively an appending operation.
In [145]:se=pd.Series([1,2,3])In [146]:seOut[146]:0 11 22 3dtype: int64In [147]:se[5]=5.In [148]:seOut[148]:0 1.01 2.02 3.05 5.0dtype: float64
ADataFrame
can be enlarged on either axis via.loc
.
In [149]:dfi=pd.DataFrame(np.arange(6).reshape(3,2), .....:columns=['A','B']) .....:In [150]:dfiOut[150]: A B0 0 11 2 32 4 5In [151]:dfi.loc[:,'C']=dfi.loc[:,'A']In [152]:dfiOut[152]: A B C0 0 1 01 2 3 22 4 5 4
This is like anappend
operation on theDataFrame
.
In [153]:dfi.loc[3]=5In [154]:dfiOut[154]: A B C0 0 1 01 2 3 22 4 5 43 5 5 5
Fast scalar value getting and setting#
Since indexing with[]
must handle a lot of cases (single-label access,slicing, boolean indexing, etc.), it has a bit of overhead in order to figureout what you’re asking for. If you only want to access a scalar value, thefastest way is to use theat
andiat
methods, which are implemented onall of the data structures.
Similarly toloc
,at
provideslabel based scalar lookups, while,iat
providesinteger based lookups analogously toiloc
In [155]:s.iat[5]Out[155]:5In [156]:df.at[dates[5],'A']Out[156]:0.1136484096888855In [157]:df.iat[3,0]Out[157]:-0.7067711336300845
You can also set using these same indexers.
In [158]:df.at[dates[5],'E']=7In [159]:df.iat[3,0]=7
at
may enlarge the object in-place as above if the indexer is missing.
In [160]:df.at[dates[-1]+pd.Timedelta('1 day'),0]=7In [161]:dfOut[161]: A B C D E 02000-01-01 -0.282863 0.469112 -1.509059 -1.135632 NaN NaN2000-01-02 -0.173215 1.212112 0.119209 -1.044236 NaN NaN2000-01-03 -2.104569 -0.861849 -0.494929 1.071804 NaN NaN2000-01-04 7.000000 0.721555 -1.039575 0.271860 NaN NaN2000-01-05 0.567020 -0.424972 0.276232 -1.087401 NaN NaN2000-01-06 0.113648 -0.673690 -1.478427 0.524988 7.0 NaN2000-01-07 0.577046 0.404705 -1.715002 -1.039268 NaN NaN2000-01-08 -1.157892 -0.370647 -1.344312 0.844885 NaN NaN2000-01-09 NaN NaN NaN NaN NaN 7.0
Boolean indexing#
Another common operation is the use of boolean vectors to filter the data.The operators are:|
foror
,&
forand
, and~
fornot
.Thesemust be grouped by using parentheses, since by default Python willevaluate an expression such asdf['A']>2&df['B']<3
asdf['A']>(2&df['B'])<3
, while the desired evaluation order is(df['A']>2)&(df['B']<3)
.
Using a boolean vector to index a Series works exactly as in a NumPy ndarray:
In [162]:s=pd.Series(range(-3,4))In [163]:sOut[163]:0 -31 -22 -13 04 15 26 3dtype: int64In [164]:s[s>0]Out[164]:4 15 26 3dtype: int64In [165]:s[(s<-1)|(s>0.5)]Out[165]:0 -31 -24 15 26 3dtype: int64In [166]:s[~(s<0)]Out[166]:3 04 15 26 3dtype: int64
You may select rows from a DataFrame using a boolean vector the same length asthe DataFrame’s index (for example, something derived from one of the columnsof the DataFrame):
In [167]:df[df['A']>0]Out[167]: A B C D E 02000-01-04 7.000000 0.721555 -1.039575 0.271860 NaN NaN2000-01-05 0.567020 -0.424972 0.276232 -1.087401 NaN NaN2000-01-06 0.113648 -0.673690 -1.478427 0.524988 7.0 NaN2000-01-07 0.577046 0.404705 -1.715002 -1.039268 NaN NaN
List comprehensions and themap
method of Series can also be used to producemore complex criteria:
In [168]:df2=pd.DataFrame({'a':['one','one','two','three','two','one','six'], .....:'b':['x','y','y','x','y','x','x'], .....:'c':np.random.randn(7)}) .....:# only want 'two' or 'three'In [169]:criterion=df2['a'].map(lambdax:x.startswith('t'))In [170]:df2[criterion]Out[170]: a b c2 two y 0.0412903 three x 0.3617194 two y -0.238075# equivalent but slowerIn [171]:df2[[x.startswith('t')forxindf2['a']]]Out[171]: a b c2 two y 0.0412903 three x 0.3617194 two y -0.238075# Multiple criteriaIn [172]:df2[criterion&(df2['b']=='x')]Out[172]: a b c3 three x 0.361719
With the choice methodsSelection by Label,Selection by Position,andAdvanced Indexing you may select along more than one axis using boolean vectors combined with other indexing expressions.
In [173]:df2.loc[criterion&(df2['b']=='x'),'b':'c']Out[173]: b c3 x 0.361719
Warning
iloc
supports two kinds of boolean indexing. If the indexer is a booleanSeries
,an error will be raised. For instance, in the following example,df.iloc[s.values,1]
is ok.The boolean indexer is an array. Butdf.iloc[s,1]
would raiseValueError
.
In [174]:df=pd.DataFrame([[1,2],[3,4],[5,6]], .....:index=list('abc'), .....:columns=['A','B']) .....:In [175]:s=(df['A']>2)In [176]:sOut[176]:a Falseb Truec TrueName: A, dtype: boolIn [177]:df.loc[s,'B']Out[177]:b 4c 6Name: B, dtype: int64In [178]:df.iloc[s.values,1]Out[178]:b 4c 6Name: B, dtype: int64
Indexing with isin#
Consider theisin()
method ofSeries
, which returns a booleanvector that is true wherever theSeries
elements exist in the passed list.This allows you to select rows where one or more columns have values you want:
In [179]:s=pd.Series(np.arange(5),index=np.arange(5)[::-1],dtype='int64')In [180]:sOut[180]:4 03 12 21 30 4dtype: int64In [181]:s.isin([2,4,6])Out[181]:4 False3 False2 True1 False0 Truedtype: boolIn [182]:s[s.isin([2,4,6])]Out[182]:2 20 4dtype: int64
The same method is available forIndex
objects and is useful for the caseswhen you don’t know which of the sought labels are in fact present:
In [183]:s[s.index.isin([2,4,6])]Out[183]:4 02 2dtype: int64# compare it to the followingIn [184]:s.reindex([2,4,6])Out[184]:2 2.04 0.06 NaNdtype: float64
In addition to that,MultiIndex
allows selecting a separate level to usein the membership check:
In [185]:s_mi=pd.Series(np.arange(6), .....:index=pd.MultiIndex.from_product([[0,1],['a','b','c']])) .....:In [186]:s_miOut[186]:0 a 0 b 1 c 21 a 3 b 4 c 5dtype: int64In [187]:s_mi.iloc[s_mi.index.isin([(1,'a'),(2,'b'),(0,'c')])]Out[187]:0 c 21 a 3dtype: int64In [188]:s_mi.iloc[s_mi.index.isin(['a','c','e'],level=1)]Out[188]:0 a 0 c 21 a 3 c 5dtype: int64
DataFrame also has anisin()
method. When callingisin
, pass a set ofvalues as either an array or dict. If values is an array,isin
returnsa DataFrame of booleans that is the same shape as the original DataFrame, with Truewherever the element is in the sequence of values.
In [189]:df=pd.DataFrame({'vals':[1,2,3,4],'ids':['a','b','f','n'], .....:'ids2':['a','n','c','n']}) .....:In [190]:values=['a','b',1,3]In [191]:df.isin(values)Out[191]: vals ids ids20 True True True1 False True False2 True False False3 False False False
Oftentimes you’ll want to match certain values with certain columns.Just make values adict
where the key is the column, and the value isa list of items you want to check for.
In [192]:values={'ids':['a','b'],'vals':[1,3]}In [193]:df.isin(values)Out[193]: vals ids ids20 True True False1 False True False2 True False False3 False False False
To return the DataFrame of booleans where the values arenot in the original DataFrame,use the~
operator:
In [194]:values={'ids':['a','b'],'vals':[1,3]}In [195]:~df.isin(values)Out[195]: vals ids ids20 False False True1 True False True2 False True True3 True True True
Combine DataFrame’sisin
with theany()
andall()
methods toquickly select subsets of your data that meet a given criteria.To select a row where each column meets its own criterion:
In [196]:values={'ids':['a','b'],'ids2':['a','c'],'vals':[1,3]}In [197]:row_mask=df.isin(values).all(1)In [198]:df[row_mask]Out[198]: vals ids ids20 1 a a
Thewhere()
Method and Masking#
Selecting values from a Series with a boolean vector generally returns asubset of the data. To guarantee that selection output has the same shape asthe original data, you can use thewhere
method inSeries
andDataFrame
.
To return only the selected rows:
In [199]:s[s>0]Out[199]:3 12 21 30 4dtype: int64
To return a Series of the same shape as the original:
In [200]:s.where(s>0)Out[200]:4 NaN3 1.02 2.01 3.00 4.0dtype: float64
Selecting values from a DataFrame with a boolean criterion now also preservesinput data shape.where
is used under the hood as the implementation.The code below is equivalent todf.where(df<0)
.
In [201]:dates=pd.date_range('1/1/2000',periods=8)In [202]:df=pd.DataFrame(np.random.randn(8,4), .....:index=dates,columns=['A','B','C','D']) .....:In [203]:df[df<0]Out[203]: A B C D2000-01-01 -2.104139 -1.309525 NaN NaN2000-01-02 -0.352480 NaN -1.192319 NaN2000-01-03 -0.864883 NaN -0.227870 NaN2000-01-04 NaN -1.222082 NaN -1.2332032000-01-05 NaN -0.605656 -1.169184 NaN2000-01-06 NaN -0.948458 NaN -0.6847182000-01-07 -2.670153 -0.114722 NaN -0.0480482000-01-08 NaN NaN -0.048788 -0.808838
In addition,where
takes an optionalother
argument for replacement ofvalues where the condition is False, in the returned copy.
In [204]:df.where(df<0,-df)Out[204]: A B C D2000-01-01 -2.104139 -1.309525 -0.485855 -0.2451662000-01-02 -0.352480 -0.390389 -1.192319 -1.6558242000-01-03 -0.864883 -0.299674 -0.227870 -0.2810592000-01-04 -0.846958 -1.222082 -0.600705 -1.2332032000-01-05 -0.669692 -0.605656 -1.169184 -0.3424162000-01-06 -0.868584 -0.948458 -2.297780 -0.6847182000-01-07 -2.670153 -0.114722 -0.168904 -0.0480482000-01-08 -0.801196 -1.392071 -0.048788 -0.808838
You may wish to set values based on some boolean criteria.This can be done intuitively like so:
In [205]:s2=s.copy()In [206]:s2[s2<0]=0In [207]:s2Out[207]:4 03 12 21 30 4dtype: int64In [208]:df2=df.copy()In [209]:df2[df2<0]=0In [210]:df2Out[210]: A B C D2000-01-01 0.000000 0.000000 0.485855 0.2451662000-01-02 0.000000 0.390389 0.000000 1.6558242000-01-03 0.000000 0.299674 0.000000 0.2810592000-01-04 0.846958 0.000000 0.600705 0.0000002000-01-05 0.669692 0.000000 0.000000 0.3424162000-01-06 0.868584 0.000000 2.297780 0.0000002000-01-07 0.000000 0.000000 0.168904 0.0000002000-01-08 0.801196 1.392071 0.000000 0.000000
where
returns a modified copy of the data.
Note
The signature forDataFrame.where()
differs fromnumpy.where()
.Roughlydf1.where(m,df2)
is equivalent tonp.where(m,df1,df2)
.
In [211]:df.where(df<0,-df)==np.where(df<0,df,-df)Out[211]: A B C D2000-01-01 True True True True2000-01-02 True True True True2000-01-03 True True True True2000-01-04 True True True True2000-01-05 True True True True2000-01-06 True True True True2000-01-07 True True True True2000-01-08 True True True True
Alignment
Furthermore,where
aligns the input boolean condition (ndarray or DataFrame),such that partial selection with setting is possible. This is analogous topartial setting via.loc
(but on the contents rather than the axis labels).
In [212]:df2=df.copy()In [213]:df2[df2[1:4]>0]=3In [214]:df2Out[214]: A B C D2000-01-01 -2.104139 -1.309525 0.485855 0.2451662000-01-02 -0.352480 3.000000 -1.192319 3.0000002000-01-03 -0.864883 3.000000 -0.227870 3.0000002000-01-04 3.000000 -1.222082 3.000000 -1.2332032000-01-05 0.669692 -0.605656 -1.169184 0.3424162000-01-06 0.868584 -0.948458 2.297780 -0.6847182000-01-07 -2.670153 -0.114722 0.168904 -0.0480482000-01-08 0.801196 1.392071 -0.048788 -0.808838
Where can also acceptaxis
andlevel
parameters to align the input whenperforming thewhere
.
In [215]:df2=df.copy()In [216]:df2.where(df2>0,df2['A'],axis='index')Out[216]: A B C D2000-01-01 -2.104139 -2.104139 0.485855 0.2451662000-01-02 -0.352480 0.390389 -0.352480 1.6558242000-01-03 -0.864883 0.299674 -0.864883 0.2810592000-01-04 0.846958 0.846958 0.600705 0.8469582000-01-05 0.669692 0.669692 0.669692 0.3424162000-01-06 0.868584 0.868584 2.297780 0.8685842000-01-07 -2.670153 -2.670153 0.168904 -2.6701532000-01-08 0.801196 1.392071 0.801196 0.801196
This is equivalent to (but faster than) the following.
In [217]:df2=df.copy()In [218]:df.apply(lambdax,y:x.where(x>0,y),y=df['A'])Out[218]: A B C D2000-01-01 -2.104139 -2.104139 0.485855 0.2451662000-01-02 -0.352480 0.390389 -0.352480 1.6558242000-01-03 -0.864883 0.299674 -0.864883 0.2810592000-01-04 0.846958 0.846958 0.600705 0.8469582000-01-05 0.669692 0.669692 0.669692 0.3424162000-01-06 0.868584 0.868584 2.297780 0.8685842000-01-07 -2.670153 -2.670153 0.168904 -2.6701532000-01-08 0.801196 1.392071 0.801196 0.801196
where
can accept a callable as condition andother
arguments. The function mustbe with one argument (the calling Series or DataFrame) and that returns valid outputas condition andother
argument.
In [219]:df3=pd.DataFrame({'A':[1,2,3], .....:'B':[4,5,6], .....:'C':[7,8,9]}) .....:In [220]:df3.where(lambdax:x>4,lambdax:x+10)Out[220]: A B C0 11 14 71 12 5 82 13 6 9
Mask#
mask()
is the inverse boolean operation ofwhere
.
In [221]:s.mask(s>=0)Out[221]:4 NaN3 NaN2 NaN1 NaN0 NaNdtype: float64In [222]:df.mask(df>=0)Out[222]: A B C D2000-01-01 -2.104139 -1.309525 NaN NaN2000-01-02 -0.352480 NaN -1.192319 NaN2000-01-03 -0.864883 NaN -0.227870 NaN2000-01-04 NaN -1.222082 NaN -1.2332032000-01-05 NaN -0.605656 -1.169184 NaN2000-01-06 NaN -0.948458 NaN -0.6847182000-01-07 -2.670153 -0.114722 NaN -0.0480482000-01-08 NaN NaN -0.048788 -0.808838
Setting with enlargement conditionally usingnumpy()
#
An alternative towhere()
is to usenumpy.where()
.Combined with setting a new column, you can use it to enlarge a DataFrame where thevalues are determined conditionally.
Consider you have two choices to choose from in the following DataFrame. And you want toset a new column color to ‘green’ when the second column has ‘Z’. You can do thefollowing:
In [223]:df=pd.DataFrame({'col1':list('ABBC'),'col2':list('ZZXY')})In [224]:df['color']=np.where(df['col2']=='Z','green','red')In [225]:dfOut[225]: col1 col2 color0 A Z green1 B Z green2 B X red3 C Y red
If you have multiple conditions, you can usenumpy.select()
to achieve that. Saycorresponding to three conditions there are three choice of colors, with a fourth coloras a fallback, you can do the following.
In [226]:conditions=[ .....:(df['col2']=='Z')&(df['col1']=='A'), .....:(df['col2']=='Z')&(df['col1']=='B'), .....:(df['col1']=='B') .....:] .....:In [227]:choices=['yellow','blue','purple']In [228]:df['color']=np.select(conditions,choices,default='black')In [229]:dfOut[229]: col1 col2 color0 A Z yellow1 B Z blue2 B X purple3 C Y black
Thequery()
Method#
DataFrame
objects have aquery()
method that allows selection using an expression.
You can get the value of the frame where columnb
has valuesbetween the values of columnsa
andc
. For example:
In [230]:n=10In [231]:df=pd.DataFrame(np.random.rand(n,3),columns=list('abc'))In [232]:dfOut[232]: a b c0 0.438921 0.118680 0.8636701 0.138138 0.577363 0.6866022 0.595307 0.564592 0.5206303 0.913052 0.926075 0.6161844 0.078718 0.854477 0.8987255 0.076404 0.523211 0.5915386 0.792342 0.216974 0.5640567 0.397890 0.454131 0.9157168 0.074315 0.437913 0.0197949 0.559209 0.502065 0.026437# pure pythonIn [233]:df[(df['a']<df['b'])&(df['b']<df['c'])]Out[233]: a b c1 0.138138 0.577363 0.6866024 0.078718 0.854477 0.8987255 0.076404 0.523211 0.5915387 0.397890 0.454131 0.915716# queryIn [234]:df.query('(a < b) & (b < c)')Out[234]: a b c1 0.138138 0.577363 0.6866024 0.078718 0.854477 0.8987255 0.076404 0.523211 0.5915387 0.397890 0.454131 0.915716
Do the same thing but fall back on a named index if there is no columnwith the namea
.
In [235]:df=pd.DataFrame(np.random.randint(n/2,size=(n,2)),columns=list('bc'))In [236]:df.index.name='a'In [237]:dfOut[237]: b ca0 0 41 0 12 3 43 4 34 1 45 0 36 0 17 3 48 2 39 1 1In [238]:df.query('a < b and b < c')Out[238]: b ca2 3 4
If instead you don’t want to or cannot name your index, you can use the nameindex
in your query expression:
In [239]:df=pd.DataFrame(np.random.randint(n,size=(n,2)),columns=list('bc'))In [240]:dfOut[240]: b c0 3 11 3 02 5 63 5 24 7 45 0 16 2 57 0 18 6 09 7 9In [241]:df.query('index < b < c')Out[241]: b c2 5 6
Note
If the name of your index overlaps with a column name, the column name isgiven precedence. For example,
In [242]:df=pd.DataFrame({'a':np.random.randint(5,size=5)})In [243]:df.index.name='a'In [244]:df.query('a > 2')# uses the column 'a', not the indexOut[244]: aa1 33 3
You can still use the index in a query expression by using the specialidentifier ‘index’:
In [245]:df.query('index > 2')Out[245]: aa3 34 2
If for some reason you have a column namedindex
, then you can refer tothe index asilevel_0
as well, but at this point you should considerrenaming your columns to something less ambiguous.
MultiIndex
query()
Syntax#
You can also use the levels of aDataFrame
with aMultiIndex
as if they were columns in the frame:
In [246]:n=10In [247]:colors=np.random.choice(['red','green'],size=n)In [248]:foods=np.random.choice(['eggs','ham'],size=n)In [249]:colorsOut[249]:array(['red', 'red', 'red', 'green', 'green', 'green', 'green', 'green', 'green', 'green'], dtype='<U5')In [250]:foodsOut[250]:array(['ham', 'ham', 'eggs', 'eggs', 'eggs', 'ham', 'ham', 'eggs', 'eggs', 'eggs'], dtype='<U4')In [251]:index=pd.MultiIndex.from_arrays([colors,foods],names=['color','food'])In [252]:df=pd.DataFrame(np.random.randn(n,2),index=index)In [253]:dfOut[253]: 0 1color foodred ham 0.194889 -0.381994 ham 0.318587 2.089075 eggs -0.728293 -0.090255green eggs -0.748199 1.318931 eggs -2.029766 0.792652 ham 0.461007 -0.542749 ham -0.305384 -0.479195 eggs 0.095031 -0.270099 eggs -0.707140 -0.773882 eggs 0.229453 0.304418In [254]:df.query('color == "red"')Out[254]: 0 1color foodred ham 0.194889 -0.381994 ham 0.318587 2.089075 eggs -0.728293 -0.090255
If the levels of theMultiIndex
are unnamed, you can refer to them usingspecial names:
In [255]:df.index.names=[None,None]In [256]:dfOut[256]: 0 1red ham 0.194889 -0.381994 ham 0.318587 2.089075 eggs -0.728293 -0.090255green eggs -0.748199 1.318931 eggs -2.029766 0.792652 ham 0.461007 -0.542749 ham -0.305384 -0.479195 eggs 0.095031 -0.270099 eggs -0.707140 -0.773882 eggs 0.229453 0.304418In [257]:df.query('ilevel_0 == "red"')Out[257]: 0 1red ham 0.194889 -0.381994 ham 0.318587 2.089075 eggs -0.728293 -0.090255
The convention isilevel_0
, which means “index level 0” for the 0th levelof theindex
.
query()
Use Cases#
A use case forquery()
is when you have a collection ofDataFrame
objects that have a subset of column names (or indexlevels/names) in common. You can pass the same query to both frameswithouthaving to specify which frame you’re interested in querying
In [258]:df=pd.DataFrame(np.random.rand(n,3),columns=list('abc'))In [259]:dfOut[259]: a b c0 0.224283 0.736107 0.1391681 0.302827 0.657803 0.7138972 0.611185 0.136624 0.9849603 0.195246 0.123436 0.6277124 0.618673 0.371660 0.0479025 0.480088 0.062993 0.1857606 0.568018 0.483467 0.4452897 0.309040 0.274580 0.5871018 0.258993 0.477769 0.3702559 0.550459 0.840870 0.304611In [260]:df2=pd.DataFrame(np.random.rand(n+2,3),columns=df.columns)In [261]:df2Out[261]: a b c0 0.357579 0.229800 0.5960011 0.309059 0.957923 0.9656632 0.123102 0.336914 0.3186163 0.526506 0.323321 0.8608134 0.518736 0.486514 0.3847245 0.190804 0.505723 0.6145336 0.891939 0.623977 0.6766397 0.480559 0.378528 0.4608588 0.420223 0.136404 0.1412959 0.732206 0.419540 0.60467510 0.604466 0.848974 0.89616511 0.589168 0.920046 0.732716In [262]:expr='0.0 <= a <= c <= 0.5'In [263]:map(lambdaframe:frame.query(expr),[df,df2])Out[263]:<map at 0x7f1056b33400>
query()
Python versus pandas Syntax Comparison#
Full numpy-like syntax:
In [264]:df=pd.DataFrame(np.random.randint(n,size=(n,3)),columns=list('abc'))In [265]:dfOut[265]: a b c0 7 8 91 1 0 72 2 7 23 6 2 24 2 6 35 3 8 26 1 7 27 5 1 58 9 8 09 1 5 0In [266]:df.query('(a < b) & (b < c)')Out[266]: a b c0 7 8 9In [267]:df[(df['a']<df['b'])&(df['b']<df['c'])]Out[267]: a b c0 7 8 9
Slightly nicer by removing the parentheses (comparison operators bind tighterthan&
and|
):
In [268]:df.query('a < b & b < c')Out[268]: a b c0 7 8 9
Use English instead of symbols:
In [269]:df.query('a < b and b < c')Out[269]: a b c0 7 8 9
Pretty close to how you might write it on paper:
In [270]:df.query('a < b < c')Out[270]: a b c0 7 8 9
Thein
andnotin
operators#
query()
also supports special use of Python’sin
andnotin
comparison operators, providing a succinct syntax for calling theisin
method of aSeries
orDataFrame
.
# get all rows where columns "a" and "b" have overlapping valuesIn [271]:df=pd.DataFrame({'a':list('aabbccddeeff'),'b':list('aaaabbbbcccc'), .....:'c':np.random.randint(5,size=12), .....:'d':np.random.randint(9,size=12)}) .....:In [272]:dfOut[272]: a b c d0 a a 2 61 a a 4 72 b a 1 63 b a 2 14 c b 3 65 c b 0 26 d b 3 37 d b 2 18 e c 4 39 e c 2 010 f c 0 611 f c 1 2In [273]:df.query('a in b')Out[273]: a b c d0 a a 2 61 a a 4 72 b a 1 63 b a 2 14 c b 3 65 c b 0 2# How you'd do it in pure PythonIn [274]:df[df['a'].isin(df['b'])]Out[274]: a b c d0 a a 2 61 a a 4 72 b a 1 63 b a 2 14 c b 3 65 c b 0 2In [275]:df.query('a not in b')Out[275]: a b c d6 d b 3 37 d b 2 18 e c 4 39 e c 2 010 f c 0 611 f c 1 2# pure PythonIn [276]:df[~df['a'].isin(df['b'])]Out[276]: a b c d6 d b 3 37 d b 2 18 e c 4 39 e c 2 010 f c 0 611 f c 1 2
You can combine this with other expressions for very succinct queries:
# rows where cols a and b have overlapping values# and col c's values are less than col d'sIn [277]:df.query('a in b and c < d')Out[277]: a b c d0 a a 2 61 a a 4 72 b a 1 64 c b 3 65 c b 0 2# pure PythonIn [278]:df[df['b'].isin(df['a'])&(df['c']<df['d'])]Out[278]: a b c d0 a a 2 61 a a 4 72 b a 1 64 c b 3 65 c b 0 210 f c 0 611 f c 1 2
Note
Note thatin
andnotin
are evaluated in Python, sincenumexpr
has no equivalent of this operation. However,only thein
/notin
expression itself is evaluated in vanilla Python. For example, in theexpression
df.query('a in b + c + d')
(b+c+d)
is evaluated bynumexpr
andthen thein
operation is evaluated in plain Python. In general, any operations that canbe evaluated usingnumexpr
will be.
Special use of the==
operator withlist
objects#
Comparing alist
of values to a column using==
/!=
works similarlytoin
/notin
.
In [279]:df.query('b == ["a", "b", "c"]')Out[279]: a b c d0 a a 2 61 a a 4 72 b a 1 63 b a 2 14 c b 3 65 c b 0 26 d b 3 37 d b 2 18 e c 4 39 e c 2 010 f c 0 611 f c 1 2# pure PythonIn [280]:df[df['b'].isin(["a","b","c"])]Out[280]: a b c d0 a a 2 61 a a 4 72 b a 1 63 b a 2 14 c b 3 65 c b 0 26 d b 3 37 d b 2 18 e c 4 39 e c 2 010 f c 0 611 f c 1 2In [281]:df.query('c == [1, 2]')Out[281]: a b c d0 a a 2 62 b a 1 63 b a 2 17 d b 2 19 e c 2 011 f c 1 2In [282]:df.query('c != [1, 2]')Out[282]: a b c d1 a a 4 74 c b 3 65 c b 0 26 d b 3 38 e c 4 310 f c 0 6# using in/not inIn [283]:df.query('[1, 2] in c')Out[283]: a b c d0 a a 2 62 b a 1 63 b a 2 17 d b 2 19 e c 2 011 f c 1 2In [284]:df.query('[1, 2] not in c')Out[284]: a b c d1 a a 4 74 c b 3 65 c b 0 26 d b 3 38 e c 4 310 f c 0 6# pure PythonIn [285]:df[df['c'].isin([1,2])]Out[285]: a b c d0 a a 2 62 b a 1 63 b a 2 17 d b 2 19 e c 2 011 f c 1 2
Boolean operators#
You can negate boolean expressions with the wordnot
or the~
operator.
In [286]:df=pd.DataFrame(np.random.rand(n,3),columns=list('abc'))In [287]:df['bools']=np.random.rand(len(df))>0.5In [288]:df.query('~bools')Out[288]: a b c bools2 0.697753 0.212799 0.329209 False7 0.275396 0.691034 0.826619 False8 0.190649 0.558748 0.262467 FalseIn [289]:df.query('not bools')Out[289]: a b c bools2 0.697753 0.212799 0.329209 False7 0.275396 0.691034 0.826619 False8 0.190649 0.558748 0.262467 FalseIn [290]:df.query('not bools')==df[~df['bools']]Out[290]: a b c bools2 True True True True7 True True True True8 True True True True
Of course, expressions can be arbitrarily complex too:
# short query syntaxIn [291]:shorter=df.query('a < b < c and (not bools) or bools > 2')# equivalent in pure PythonIn [292]:longer=df[(df['a']<df['b']) .....:&(df['b']<df['c']) .....:&(~df['bools']) .....:|(df['bools']>2)] .....:In [293]:shorterOut[293]: a b c bools7 0.275396 0.691034 0.826619 FalseIn [294]:longerOut[294]: a b c bools7 0.275396 0.691034 0.826619 FalseIn [295]:shorter==longerOut[295]: a b c bools7 True True True True
Performance ofquery()
#
DataFrame.query()
usingnumexpr
is slightly faster than Python forlarge frames.
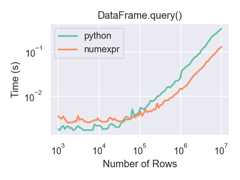
You will only see the performance benefits of using thenumexpr
enginewithDataFrame.query()
if your frame has more than approximately 100,000rows.
This plot was created using aDataFrame
with 3 columns each containingfloating point values generated usingnumpy.random.randn()
.
In [296]:df=pd.DataFrame(np.random.randn(8,4), .....:index=dates,columns=['A','B','C','D']) .....:In [297]:df2=df.copy()
Duplicate data#
If you want to identify and remove duplicate rows in a DataFrame, there aretwo methods that will help:duplicated
anddrop_duplicates
. Eachtakes as an argument the columns to use to identify duplicated rows.
duplicated
returns a boolean vector whose length is the number of rows, and which indicates whether a row is duplicated.drop_duplicates
removes duplicate rows.
By default, the first observed row of a duplicate set is considered unique, buteach method has akeep
parameter to specify targets to be kept.
keep='first'
(default): mark / drop duplicates except for the first occurrence.keep='last'
: mark / drop duplicates except for the last occurrence.keep=False
: mark / drop all duplicates.
In [298]:df2=pd.DataFrame({'a':['one','one','two','two','two','three','four'], .....:'b':['x','y','x','y','x','x','x'], .....:'c':np.random.randn(7)}) .....:In [299]:df2Out[299]: a b c0 one x -1.0671371 one y 0.3095002 two x -0.2110563 two y -1.8420234 two x -0.3908205 three x -1.9644756 four x 1.298329In [300]:df2.duplicated('a')Out[300]:0 False1 True2 False3 True4 True5 False6 Falsedtype: boolIn [301]:df2.duplicated('a',keep='last')Out[301]:0 True1 False2 True3 True4 False5 False6 Falsedtype: boolIn [302]:df2.duplicated('a',keep=False)Out[302]:0 True1 True2 True3 True4 True5 False6 Falsedtype: boolIn [303]:df2.drop_duplicates('a')Out[303]: a b c0 one x -1.0671372 two x -0.2110565 three x -1.9644756 four x 1.298329In [304]:df2.drop_duplicates('a',keep='last')Out[304]: a b c1 one y 0.3095004 two x -0.3908205 three x -1.9644756 four x 1.298329In [305]:df2.drop_duplicates('a',keep=False)Out[305]: a b c5 three x -1.9644756 four x 1.298329
Also, you can pass a list of columns to identify duplications.
In [306]:df2.duplicated(['a','b'])Out[306]:0 False1 False2 False3 False4 True5 False6 Falsedtype: boolIn [307]:df2.drop_duplicates(['a','b'])Out[307]: a b c0 one x -1.0671371 one y 0.3095002 two x -0.2110563 two y -1.8420235 three x -1.9644756 four x 1.298329
To drop duplicates by index value, useIndex.duplicated
then perform slicing.The same set of options are available for thekeep
parameter.
In [308]:df3=pd.DataFrame({'a':np.arange(6), .....:'b':np.random.randn(6)}, .....:index=['a','a','b','c','b','a']) .....:In [309]:df3Out[309]: a ba 0 1.440455a 1 2.456086b 2 1.038402c 3 -0.894409b 4 0.683536a 5 3.082764In [310]:df3.index.duplicated()Out[310]:array([False, True, False, False, True, True])In [311]:df3[~df3.index.duplicated()]Out[311]: a ba 0 1.440455b 2 1.038402c 3 -0.894409In [312]:df3[~df3.index.duplicated(keep='last')]Out[312]: a bc 3 -0.894409b 4 0.683536a 5 3.082764In [313]:df3[~df3.index.duplicated(keep=False)]Out[313]: a bc 3 -0.894409
Dictionary-likeget()
method#
Each of Series or DataFrame have aget
method which can return adefault value.
In [314]:s=pd.Series([1,2,3],index=['a','b','c'])In [315]:s.get('a')# equivalent to s['a']Out[315]:1In [316]:s.get('x',default=-1)Out[316]:-1
Looking up values by index/column labels#
Sometimes you want to extract a set of values given a sequence of row labelsand column labels, this can be achieved bypandas.factorize
and NumPy indexing.For instance:
In [317]:df=pd.DataFrame({'col':["A","A","B","B"], .....:'A':[80,23,np.nan,22], .....:'B':[80,55,76,67]}) .....:In [318]:dfOut[318]: col A B0 A 80.0 801 A 23.0 552 B NaN 763 B 22.0 67In [319]:idx,cols=pd.factorize(df['col'])In [320]:df.reindex(cols,axis=1).to_numpy()[np.arange(len(df)),idx]Out[320]:array([80., 23., 76., 67.])
Formerly this could be achieved with the dedicatedDataFrame.lookup
methodwhich was deprecated in version 1.2.0 and removed in version 2.0.0.
Index objects#
The pandasIndex
class and its subclasses can be viewed asimplementing anordered multiset. Duplicates are allowed.
Index
also provides the infrastructure necessary forlookups, data alignment, and reindexing. The easiest way to create anIndex
directly is to pass alist
or other sequence toIndex
:
In [321]:index=pd.Index(['e','d','a','b'])In [322]:indexOut[322]:Index(['e', 'd', 'a', 'b'], dtype='object')In [323]:'d'inindexOut[323]:True
or using numbers:
In [324]:index=pd.Index([1,5,12])In [325]:indexOut[325]:Index([1, 5, 12], dtype='int64')In [326]:5inindexOut[326]:True
If no dtype is given,Index
tries to infer the dtype from the data.It is also possible to give an explicit dtype when instantiating anIndex
:
In [327]:index=pd.Index(['e','d','a','b'],dtype="string")In [328]:indexOut[328]:Index(['e', 'd', 'a', 'b'], dtype='string')In [329]:index=pd.Index([1,5,12],dtype="int8")In [330]:indexOut[330]:Index([1, 5, 12], dtype='int8')In [331]:index=pd.Index([1,5,12],dtype="float32")In [332]:indexOut[332]:Index([1.0, 5.0, 12.0], dtype='float32')
You can also pass aname
to be stored in the index:
In [333]:index=pd.Index(['e','d','a','b'],name='something')In [334]:index.nameOut[334]:'something'
The name, if set, will be shown in the console display:
In [335]:index=pd.Index(list(range(5)),name='rows')In [336]:columns=pd.Index(['A','B','C'],name='cols')In [337]:df=pd.DataFrame(np.random.randn(5,3),index=index,columns=columns)In [338]:dfOut[338]:cols A B Crows0 1.295989 -1.051694 1.3404291 -2.366110 0.428241 0.3872752 0.433306 0.929548 0.2780943 2.154730 -0.315628 0.2642234 1.126818 1.132290 -0.353310In [339]:df['A']Out[339]:rows0 1.2959891 -2.3661102 0.4333063 2.1547304 1.126818Name: A, dtype: float64
Setting metadata#
Indexes are “mostly immutable”, but it is possible to set and change theirname
attribute. You can use therename
,set_names
to set these attributesdirectly, and they default to returning a copy.
SeeAdvanced Indexing for usage of MultiIndexes.
In [340]:ind=pd.Index([1,2,3])In [341]:ind.rename("apple")Out[341]:Index([1, 2, 3], dtype='int64', name='apple')In [342]:indOut[342]:Index([1, 2, 3], dtype='int64')In [343]:ind=ind.set_names(["apple"])In [344]:ind.name="bob"In [345]:indOut[345]:Index([1, 2, 3], dtype='int64', name='bob')
set_names
,set_levels
, andset_codes
also take an optionallevel
argument
In [346]:index=pd.MultiIndex.from_product([range(3),['one','two']],names=['first','second'])In [347]:indexOut[347]:MultiIndex([(0, 'one'), (0, 'two'), (1, 'one'), (1, 'two'), (2, 'one'), (2, 'two')], names=['first', 'second'])In [348]:index.levels[1]Out[348]:Index(['one', 'two'], dtype='object', name='second')In [349]:index.set_levels(["a","b"],level=1)Out[349]:MultiIndex([(0, 'a'), (0, 'b'), (1, 'a'), (1, 'b'), (2, 'a'), (2, 'b')], names=['first', 'second'])
Set operations on Index objects#
The two main operations areunion
andintersection
.Difference is provided via the.difference()
method.
In [350]:a=pd.Index(['c','b','a'])In [351]:b=pd.Index(['c','e','d'])In [352]:a.difference(b)Out[352]:Index(['a', 'b'], dtype='object')
Also available is thesymmetric_difference
operation, which returns elementsthat appear in eitheridx1
oridx2
, but not in both. This isequivalent to the Index created byidx1.difference(idx2).union(idx2.difference(idx1))
,with duplicates dropped.
In [353]:idx1=pd.Index([1,2,3,4])In [354]:idx2=pd.Index([2,3,4,5])In [355]:idx1.symmetric_difference(idx2)Out[355]:Index([1, 5], dtype='int64')
Note
The resulting index from a set operation will be sorted in ascending order.
When performingIndex.union()
between indexes with different dtypes, the indexesmust be cast to a common dtype. Typically, though not always, this is object dtype. Theexception is when performing a union between integer and float data. In this case, theinteger values are converted to float
In [356]:idx1=pd.Index([0,1,2])In [357]:idx2=pd.Index([0.5,1.5])In [358]:idx1.union(idx2)Out[358]:Index([0.0, 0.5, 1.0, 1.5, 2.0], dtype='float64')
Missing values#
Important
Even thoughIndex
can hold missing values (NaN
), it should be avoidedif you do not want any unexpected results. For example, some operationsexclude missing values implicitly.
Index.fillna
fills missing values with specified scalar value.
In [359]:idx1=pd.Index([1,np.nan,3,4])In [360]:idx1Out[360]:Index([1.0, nan, 3.0, 4.0], dtype='float64')In [361]:idx1.fillna(2)Out[361]:Index([1.0, 2.0, 3.0, 4.0], dtype='float64')In [362]:idx2=pd.DatetimeIndex([pd.Timestamp('2011-01-01'), .....:pd.NaT, .....:pd.Timestamp('2011-01-03')]) .....:In [363]:idx2Out[363]:DatetimeIndex(['2011-01-01', 'NaT', '2011-01-03'], dtype='datetime64[ns]', freq=None)In [364]:idx2.fillna(pd.Timestamp('2011-01-02'))Out[364]:DatetimeIndex(['2011-01-01', '2011-01-02', '2011-01-03'], dtype='datetime64[ns]', freq=None)
Set / reset index#
Occasionally you will load or create a data set into a DataFrame and want toadd an index after you’ve already done so. There are a couple of differentways.
Set an index#
DataFrame has aset_index()
method which takes a column name(for a regularIndex
) or a list of column names (for aMultiIndex
).To create a new, re-indexed DataFrame:
In [365]:data=pd.DataFrame({'a':['bar','bar','foo','foo'], .....:'b':['one','two','one','two'], .....:'c':['z','y','x','w'], .....:'d':[1.,2.,3,4]}) .....:In [366]:dataOut[366]: a b c d0 bar one z 1.01 bar two y 2.02 foo one x 3.03 foo two w 4.0In [367]:indexed1=data.set_index('c')In [368]:indexed1Out[368]: a b dcz bar one 1.0y bar two 2.0x foo one 3.0w foo two 4.0In [369]:indexed2=data.set_index(['a','b'])In [370]:indexed2Out[370]: c da bbar one z 1.0 two y 2.0foo one x 3.0 two w 4.0
Theappend
keyword option allow you to keep the existing index and appendthe given columns to a MultiIndex:
In [371]:frame=data.set_index('c',drop=False)In [372]:frame=frame.set_index(['a','b'],append=True)In [373]:frameOut[373]: c dc a bz bar one z 1.0y bar two y 2.0x foo one x 3.0w foo two w 4.0
Other options inset_index
allow you not drop the index columns.
In [374]:data.set_index('c',drop=False)Out[374]: a b c dcz bar one z 1.0y bar two y 2.0x foo one x 3.0w foo two w 4.0
Reset the index#
As a convenience, there is a new function on DataFrame calledreset_index()
which transfers the index values into theDataFrame’s columns and sets a simple integer index.This is the inverse operation ofset_index()
.
In [375]:dataOut[375]: a b c d0 bar one z 1.01 bar two y 2.02 foo one x 3.03 foo two w 4.0In [376]:data.reset_index()Out[376]: index a b c d0 0 bar one z 1.01 1 bar two y 2.02 2 foo one x 3.03 3 foo two w 4.0
The output is more similar to a SQL table or a record array. The names for thecolumns derived from the index are the ones stored in thenames
attribute.
You can use thelevel
keyword to remove only a portion of the index:
In [377]:frameOut[377]: c dc a bz bar one z 1.0y bar two y 2.0x foo one x 3.0w foo two w 4.0In [378]:frame.reset_index(level=1)Out[378]: a c dc bz one bar z 1.0y two bar y 2.0x one foo x 3.0w two foo w 4.0
reset_index
takes an optional parameterdrop
which if true simplydiscards the index, instead of putting index values in the DataFrame’s columns.
Adding an ad hoc index#
You can assign a custom index to theindex
attribute:
In [379]:df_idx=pd.DataFrame(range(4))In [380]:df_idx.index=pd.Index([10,20,30,40],name="a")In [381]:df_idxOut[381]: 0a10 020 130 240 3
Returning a view versus a copy#
Warning
Copy-on-Writewill become the new default in pandas 3.0. This means that chained indexing willnever work. As a consequence, theSettingWithCopyWarning
won’t be necessaryanymore.Seethis sectionfor more context.We recommend turning Copy-on-Write on to leverage the improvements with
`pd.options.mode.copy_on_write=True`
even before pandas 3.0 is available.
When setting values in a pandas object, care must be taken to avoid what is calledchainedindexing
. Here is an example.
In [382]:dfmi=pd.DataFrame([list('abcd'), .....:list('efgh'), .....:list('ijkl'), .....:list('mnop')], .....:columns=pd.MultiIndex.from_product([['one','two'], .....:['first','second']])) .....:In [383]:dfmiOut[383]: one two first second first second0 a b c d1 e f g h2 i j k l3 m n o p
Compare these two access methods:
In [384]:dfmi['one']['second']Out[384]:0 b1 f2 j3 nName: second, dtype: object
In [385]:dfmi.loc[:,('one','second')]Out[385]:0 b1 f2 j3 nName: (one, second), dtype: object
These both yield the same results, so which should you use? It is instructive to understand the orderof operations on these and why method 2 (.loc
) is much preferred over method 1 (chained[]
).
dfmi['one']
selects the first level of the columns and returns a DataFrame that is singly-indexed.Then another Python operationdfmi_with_one['second']
selects the series indexed by'second'
.This is indicated by the variabledfmi_with_one
because pandas sees these operations as separate events.e.g. separate calls to__getitem__
, so it has to treat them as linear operations, they happen one after another.
Contrast this todf.loc[:,('one','second')]
which passes a nested tuple of(slice(None),('one','second'))
to a single call to__getitem__
. This allows pandas to deal with this as a single entity. Furthermore this order of operationscan be significantlyfaster, and allows one to indexboth axes if so desired.
Why does assignment fail when using chained indexing?#
Warning
Copy-on-Writewill become the new default in pandas 3.0. This means than chained indexing willnever work. As a consequence, theSettingWithCopyWarning
won’t be necessaryanymore.Seethis sectionfor more context.We recommend turning Copy-on-Write on to leverage the improvements with
`pd.options.mode.copy_on_write=True`
even before pandas 3.0 is available.
The problem in the previous section is just a performance issue. What’s up withtheSettingWithCopy
warning? We don’tusually throw warnings around whenyou do something that might cost a few extra milliseconds!
But it turns out that assigning to the product of chained indexing hasinherently unpredictable results. To see this, think about how the Pythoninterpreter executes this code:
dfmi.loc[:,('one','second')]=value# becomesdfmi.loc.__setitem__((slice(None),('one','second')),value)
But this code is handled differently:
dfmi['one']['second']=value# becomesdfmi.__getitem__('one').__setitem__('second',value)
See that__getitem__
in there? Outside of simple cases, it’s very hard topredict whether it will return a view or a copy (it depends on the memory layoutof the array, about which pandas makes no guarantees), and therefore whetherthe__setitem__
will modifydfmi
or a temporary object that gets thrownout immediately afterward.That’s whatSettingWithCopy
is warning youabout!
Note
You may be wondering whether we should be concerned about theloc
property in the first example. Butdfmi.loc
is guaranteed to bedfmi
itself with modified indexing behavior, sodfmi.loc.__getitem__
/dfmi.loc.__setitem__
operate ondfmi
directly. Of course,dfmi.loc.__getitem__(idx)
may be a view or a copy ofdfmi
.
Sometimes aSettingWithCopy
warning will arise at times when there’s noobvious chained indexing going on.These are the bugs thatSettingWithCopy
is designed to catch! pandas is probably trying to warn youthat you’ve done this:
defdo_something(df):foo=df[['bar','baz']]# Is foo a view? A copy? Nobody knows!# ... many lines here ...# We don't know whether this will modify df or not!foo['quux']=valuereturnfoo
Yikes!
Evaluation order matters#
Warning
Copy-on-Writewill become the new default in pandas 3.0. This means than chained indexing willnever work. As a consequence, theSettingWithCopyWarning
won’t be necessaryanymore.Seethis sectionfor more context.We recommend turning Copy-on-Write on to leverage the improvements with
`pd.options.mode.copy_on_write=True`
even before pandas 3.0 is available.
When you use chained indexing, the order and type of the indexing operationpartially determine whether the result is a slice into the original object, ora copy of the slice.
pandas has theSettingWithCopyWarning
because assigning to a copy of aslice is frequently not intentional, but a mistake caused by chained indexingreturning a copy where a slice was expected.
If you would like pandas to be more or less trusting about assignment to achained indexing expression, you can set theoptionmode.chained_assignment
to one of these values:
'warn'
, the default, means aSettingWithCopyWarning
is printed.'raise'
means pandas will raise aSettingWithCopyError
you have to deal with.None
will suppress the warnings entirely.
In [386]:dfb=pd.DataFrame({'a':['one','one','two', .....:'three','two','one','six'], .....:'c':np.arange(7)}) .....:# This will show the SettingWithCopyWarning# but the frame values will be setIn [387]:dfb['c'][dfb['a'].str.startswith('o')]=42
This however is operating on a copy and will not work.
In [388]:withpd.option_context('mode.chained_assignment','warn'): .....:dfb[dfb['a'].str.startswith('o')]['c']=42 .....:
A chained assignment can also crop up in setting in a mixed dtype frame.
Note
These setting rules apply to all of.loc/.iloc
.
The following is the recommended access method using.loc
for multiple items (usingmask
) and a single item using a fixed index:
In [389]:dfc=pd.DataFrame({'a':['one','one','two', .....:'three','two','one','six'], .....:'c':np.arange(7)}) .....:In [390]:dfd=dfc.copy()# Setting multiple items using a maskIn [391]:mask=dfd['a'].str.startswith('o')In [392]:dfd.loc[mask,'c']=42In [393]:dfdOut[393]: a c0 one 421 one 422 two 23 three 34 two 45 one 426 six 6# Setting a single itemIn [394]:dfd=dfc.copy()In [395]:dfd.loc[2,'a']=11In [396]:dfdOut[396]: a c0 one 01 one 12 11 23 three 34 two 45 one 56 six 6
The followingcan work at times, but it is not guaranteed to, and therefore should be avoided:
In [397]:dfd=dfc.copy()In [398]:dfd['a'][2]=111In [399]:dfdOut[399]: a c0 one 01 one 12 111 23 three 34 two 45 one 56 six 6
Last, the subsequent example willnot work at all, and so should be avoided:
In [400]:withpd.option_context('mode.chained_assignment','raise'): .....:dfd.loc[0]['a']=1111 .....:---------------------------------------------------------------------------SettingWithCopyErrorTraceback (most recent call last)<ipython-input-400-32ce785aaa5b> in?()1withpd.option_context('mode.chained_assignment','raise'):---->2dfd.loc[0]['a']=1111~/work/pandas/pandas/pandas/core/series.py in?(self, key, value)1293)12941295check_dict_or_set_indexers(key)1296key=com.apply_if_callable(key,self)->1297cacher_needs_updating=self._check_is_chained_assignment_possible()12981299ifkeyisEllipsis:1300key=slice(None)~/work/pandas/pandas/pandas/core/series.py in?(self)1498ref=self._get_cacher()1499ifrefisnotNoneandref._is_mixed_type:1500self._check_setitem_copy(t="referent",force=True)1501returnTrue->1502returnsuper()._check_is_chained_assignment_possible()~/work/pandas/pandas/pandas/core/generic.py in?(self)4414single-dtypemeaningthatthecachershouldbeupdatedfollowing4415setting.4416"""4417 if self._is_copy:->4418 self._check_setitem_copy(t="referent")4419 return False~/work/pandas/pandas/pandas/core/generic.py in?(self, t, force)4488 "indexing.html#returning-a-view-versus-a-copy"4489 )44904491 if value == "raise":->4492 raise SettingWithCopyError(t)4493 if value == "warn":4494 warnings.warn(t, SettingWithCopyWarning, stacklevel=find_stack_level())SettingWithCopyError:A value is trying to be set on a copy of a slice from a DataFrameSee the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
Warning
The chained assignment warnings / exceptions are aiming to inform the user of a possibly invalidassignment. There may be false positives; situations where a chained assignment is inadvertentlyreported.
- Different choices for indexing
- Basics
- Attribute access
- Slicing ranges
- Selection by label
- Selection by position
- Selection by callable
- Combining positional and label-based indexing
- Selecting random samples
- Setting with enlargement
- Fast scalar value getting and setting
- Boolean indexing
- Indexing with isin
- The
where()
Method and Masking - Setting with enlargement conditionally using
numpy()
- The
query()
Method - Duplicate data
- Dictionary-like
get()
method - Looking up values by index/column labels
- Index objects
- Set / reset index
- Returning a view versus a copy