pathlib
— Object-oriented filesystem paths¶
Added in version 3.4.
Source code:Lib/pathlib/
This module offers classes representing filesystem paths with semanticsappropriate for different operating systems. Path classes are dividedbetweenpure paths, which provide purely computationaloperations without I/O, andconcrete paths, whichinherit from pure paths but also provide I/O operations.
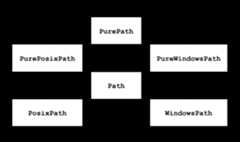
If you’ve never used this module before or just aren’t sure which class isright for your task,Path
is most likely what you need. It instantiatesaconcrete path for the platform the code is running on.
Pure paths are useful in some special cases; for example:
If you want to manipulate Windows paths on a Unix machine (or vice versa).You cannot instantiate a
WindowsPath
when running on Unix, but youcan instantiatePureWindowsPath
.You want to make sure that your code only manipulates paths without actuallyaccessing the OS. In this case, instantiating one of the pure classes may beuseful since those simply don’t have any OS-accessing operations.
See also
PEP 428: The pathlib module – object-oriented filesystem paths.
See also
For low-level path manipulation on strings, you can also use theos.path
module.
Basic use¶
Importing the main class:
>>>frompathlibimportPath
Listing subdirectories:
>>>p=Path('.')>>>[xforxinp.iterdir()ifx.is_dir()][PosixPath('.hg'), PosixPath('docs'), PosixPath('dist'), PosixPath('__pycache__'), PosixPath('build')]
Listing Python source files in this directory tree:
>>>list(p.glob('**/*.py'))[PosixPath('test_pathlib.py'), PosixPath('setup.py'), PosixPath('pathlib.py'), PosixPath('docs/conf.py'), PosixPath('build/lib/pathlib.py')]
Navigating inside a directory tree:
>>>p=Path('/etc')>>>q=p/'init.d'/'reboot'>>>qPosixPath('/etc/init.d/reboot')>>>q.resolve()PosixPath('/etc/rc.d/init.d/halt')
Querying path properties:
>>>q.exists()True>>>q.is_dir()False
Opening a file:
>>>withq.open()asf:f.readline()...'#!/bin/bash\n'
Exceptions¶
- exceptionpathlib.UnsupportedOperation¶
An exception inheriting
NotImplementedError
that is raised when anunsupported operation is called on a path object.Added in version 3.13.
Pure paths¶
Pure path objects provide path-handling operations which don’t actuallyaccess a filesystem. There are three ways to access these classes, whichwe also callflavours:
- classpathlib.PurePath(*pathsegments)¶
A generic class that represents the system’s path flavour (instantiatingit creates either a
PurePosixPath
or aPureWindowsPath
):>>>PurePath('setup.py')# Running on a Unix machinePurePosixPath('setup.py')
Each element ofpathsegments can be either a string representing apath segment, or an object implementing the
os.PathLike
interfacewhere the__fspath__()
method returns a string,such as another path object:>>>PurePath('foo','some/path','bar')PurePosixPath('foo/some/path/bar')>>>PurePath(Path('foo'),Path('bar'))PurePosixPath('foo/bar')
Whenpathsegments is empty, the current directory is assumed:
>>>PurePath()PurePosixPath('.')
If a segment is an absolute path, all previous segments are ignored(like
os.path.join()
):>>>PurePath('/etc','/usr','lib64')PurePosixPath('/usr/lib64')>>>PureWindowsPath('c:/Windows','d:bar')PureWindowsPath('d:bar')
On Windows, the drive is not reset when a rooted relative pathsegment (e.g.,
r'\foo'
) is encountered:>>>PureWindowsPath('c:/Windows','/Program Files')PureWindowsPath('c:/Program Files')
Spurious slashes and single dots are collapsed, but double dots (
'..'
)and leading double slashes ('//'
) are not, since this would change themeaning of a path for various reasons (e.g. symbolic links, UNC paths):>>>PurePath('foo//bar')PurePosixPath('foo/bar')>>>PurePath('//foo/bar')PurePosixPath('//foo/bar')>>>PurePath('foo/./bar')PurePosixPath('foo/bar')>>>PurePath('foo/../bar')PurePosixPath('foo/../bar')
(a naïve approach would make
PurePosixPath('foo/../bar')
equivalenttoPurePosixPath('bar')
, which is wrong iffoo
is a symbolic linkto another directory)Pure path objects implement the
os.PathLike
interface, allowing themto be used anywhere the interface is accepted.Changed in version 3.6:Added support for the
os.PathLike
interface.
- classpathlib.PurePosixPath(*pathsegments)¶
A subclass of
PurePath
, this path flavour represents non-Windowsfilesystem paths:>>>PurePosixPath('/etc/hosts')PurePosixPath('/etc/hosts')
pathsegments is specified similarly to
PurePath
.
- classpathlib.PureWindowsPath(*pathsegments)¶
A subclass of
PurePath
, this path flavour represents Windowsfilesystem paths, includingUNC paths:>>>PureWindowsPath('c:/','Users','Ximénez')PureWindowsPath('c:/Users/Ximénez')>>>PureWindowsPath('//server/share/file')PureWindowsPath('//server/share/file')
pathsegments is specified similarly to
PurePath
.
Regardless of the system you’re running on, you can instantiate all ofthese classes, since they don’t provide any operation that does system calls.
General properties¶
Paths are immutable andhashable. Paths of a same flavour are comparableand orderable. These properties respect the flavour’s case-foldingsemantics:
>>>PurePosixPath('foo')==PurePosixPath('FOO')False>>>PureWindowsPath('foo')==PureWindowsPath('FOO')True>>>PureWindowsPath('FOO')in{PureWindowsPath('foo')}True>>>PureWindowsPath('C:')<PureWindowsPath('d:')True
Paths of a different flavour compare unequal and cannot be ordered:
>>>PureWindowsPath('foo')==PurePosixPath('foo')False>>>PureWindowsPath('foo')<PurePosixPath('foo')Traceback (most recent call last): File"<stdin>", line1, in<module>TypeError:'<' not supported between instances of 'PureWindowsPath' and 'PurePosixPath'
Operators¶
The slash operator helps create child paths, likeos.path.join()
.If the argument is an absolute path, the previous path is ignored.On Windows, the drive is not reset when the argument is a rootedrelative path (e.g.,r'\foo'
):
>>>p=PurePath('/etc')>>>pPurePosixPath('/etc')>>>p/'init.d'/'apache2'PurePosixPath('/etc/init.d/apache2')>>>q=PurePath('bin')>>>'/usr'/qPurePosixPath('/usr/bin')>>>p/'/an_absolute_path'PurePosixPath('/an_absolute_path')>>>PureWindowsPath('c:/Windows','/Program Files')PureWindowsPath('c:/Program Files')
A path object can be used anywhere an object implementingos.PathLike
is accepted:
>>>importos>>>p=PurePath('/etc')>>>os.fspath(p)'/etc'
The string representation of a path is the raw filesystem path itself(in native form, e.g. with backslashes under Windows), which you canpass to any function taking a file path as a string:
>>>p=PurePath('/etc')>>>str(p)'/etc'>>>p=PureWindowsPath('c:/Program Files')>>>str(p)'c:\\Program Files'
Similarly, callingbytes
on a path gives the raw filesystem path as abytes object, as encoded byos.fsencode()
:
>>>bytes(p)b'/etc'
Note
Callingbytes
is only recommended under Unix. Under Windows,the unicode form is the canonical representation of filesystem paths.
Accessing individual parts¶
To access the individual “parts” (components) of a path, use the followingproperty:
- PurePath.parts¶
A tuple giving access to the path’s various components:
>>>p=PurePath('/usr/bin/python3')>>>p.parts('/', 'usr', 'bin', 'python3')>>>p=PureWindowsPath('c:/Program Files/PSF')>>>p.parts('c:\\', 'Program Files', 'PSF')
(note how the drive and local root are regrouped in a single part)
Methods and properties¶
Pure paths provide the following methods and properties:
- PurePath.parser¶
The implementation of the
os.path
module used for low-level pathparsing and joining: eitherposixpath
orntpath
.Added in version 3.13.
- PurePath.drive¶
A string representing the drive letter or name, if any:
>>>PureWindowsPath('c:/Program Files/').drive'c:'>>>PureWindowsPath('/Program Files/').drive''>>>PurePosixPath('/etc').drive''
UNC shares are also considered drives:
>>>PureWindowsPath('//host/share/foo.txt').drive'\\\\host\\share'
- PurePath.root¶
A string representing the (local or global) root, if any:
>>>PureWindowsPath('c:/Program Files/').root'\\'>>>PureWindowsPath('c:Program Files/').root''>>>PurePosixPath('/etc').root'/'
UNC shares always have a root:
>>>PureWindowsPath('//host/share').root'\\'
If the path starts with more than two successive slashes,
PurePosixPath
collapses them:>>>PurePosixPath('//etc').root'//'>>>PurePosixPath('///etc').root'/'>>>PurePosixPath('////etc').root'/'
Note
This behavior conforms toThe Open Group Base Specifications Issue 6,paragraph4.11 Pathname Resolution:
“A pathname that begins with two successive slashes may be interpreted inan implementation-defined manner, although more than two leading slashesshall be treated as a single slash.”
- PurePath.anchor¶
The concatenation of the drive and root:
>>>PureWindowsPath('c:/Program Files/').anchor'c:\\'>>>PureWindowsPath('c:Program Files/').anchor'c:'>>>PurePosixPath('/etc').anchor'/'>>>PureWindowsPath('//host/share').anchor'\\\\host\\share\\'
- PurePath.parents¶
An immutable sequence providing access to the logical ancestors ofthe path:
>>>p=PureWindowsPath('c:/foo/bar/setup.py')>>>p.parents[0]PureWindowsPath('c:/foo/bar')>>>p.parents[1]PureWindowsPath('c:/foo')>>>p.parents[2]PureWindowsPath('c:/')
Changed in version 3.10:The parents sequence now supportsslices and negative index values.
- PurePath.parent¶
The logical parent of the path:
>>>p=PurePosixPath('/a/b/c/d')>>>p.parentPurePosixPath('/a/b/c')
You cannot go past an anchor, or empty path:
>>>p=PurePosixPath('/')>>>p.parentPurePosixPath('/')>>>p=PurePosixPath('.')>>>p.parentPurePosixPath('.')
Note
This is a purely lexical operation, hence the following behaviour:
>>>p=PurePosixPath('foo/..')>>>p.parentPurePosixPath('foo')
If you want to walk an arbitrary filesystem path upwards, it isrecommended to first call
Path.resolve()
so as to resolvesymlinks and eliminate".."
components.
- PurePath.name¶
A string representing the final path component, excluding the drive androot, if any:
>>>PurePosixPath('my/library/setup.py').name'setup.py'
UNC drive names are not considered:
>>>PureWindowsPath('//some/share/setup.py').name'setup.py'>>>PureWindowsPath('//some/share').name''
- PurePath.suffix¶
The last dot-separated portion of the final component, if any:
>>>PurePosixPath('my/library/setup.py').suffix'.py'>>>PurePosixPath('my/library.tar.gz').suffix'.gz'>>>PurePosixPath('my/library').suffix''
This is commonly called the file extension.
- PurePath.suffixes¶
A list of the path’s suffixes, often called file extensions:
>>>PurePosixPath('my/library.tar.gar').suffixes['.tar', '.gar']>>>PurePosixPath('my/library.tar.gz').suffixes['.tar', '.gz']>>>PurePosixPath('my/library').suffixes[]
- PurePath.stem¶
The final path component, without its suffix:
>>>PurePosixPath('my/library.tar.gz').stem'library.tar'>>>PurePosixPath('my/library.tar').stem'library'>>>PurePosixPath('my/library').stem'library'
- PurePath.as_posix()¶
Return a string representation of the path with forward slashes (
/
):>>>p=PureWindowsPath('c:\\windows')>>>str(p)'c:\\windows'>>>p.as_posix()'c:/windows'
- PurePath.is_absolute()¶
Return whether the path is absolute or not. A path is considered absoluteif it has both a root and (if the flavour allows) a drive:
>>>PurePosixPath('/a/b').is_absolute()True>>>PurePosixPath('a/b').is_absolute()False>>>PureWindowsPath('c:/a/b').is_absolute()True>>>PureWindowsPath('/a/b').is_absolute()False>>>PureWindowsPath('c:').is_absolute()False>>>PureWindowsPath('//some/share').is_absolute()True
- PurePath.is_relative_to(other)¶
Return whether or not this path is relative to theother path.
>>>p=PurePath('/etc/passwd')>>>p.is_relative_to('/etc')True>>>p.is_relative_to('/usr')False
This method is string-based; it neither accesses the filesystem nor treats“
..
” segments specially. The following code is equivalent:>>>u=PurePath('/usr')>>>u==poruinp.parentsFalse
Added in version 3.9.
Deprecated since version 3.12, will be removed in version 3.14:Passing additional arguments is deprecated; if supplied, they are joinedwithother.
- PurePath.is_reserved()¶
With
PureWindowsPath
, returnTrue
if the path is consideredreserved under Windows,False
otherwise. WithPurePosixPath
,False
is always returned.Changed in version 3.13:Windows path names that contain a colon, or end with a dot or a space,are considered reserved. UNC paths may be reserved.
Deprecated since version 3.13, will be removed in version 3.15:This method is deprecated; use
os.path.isreserved()
to detectreserved paths on Windows.
- PurePath.joinpath(*pathsegments)¶
Calling this method is equivalent to combining the path with each ofthe givenpathsegments in turn:
>>>PurePosixPath('/etc').joinpath('passwd')PurePosixPath('/etc/passwd')>>>PurePosixPath('/etc').joinpath(PurePosixPath('passwd'))PurePosixPath('/etc/passwd')>>>PurePosixPath('/etc').joinpath('init.d','apache2')PurePosixPath('/etc/init.d/apache2')>>>PureWindowsPath('c:').joinpath('/Program Files')PureWindowsPath('c:/Program Files')
- PurePath.full_match(pattern,*,case_sensitive=None)¶
Match this path against the provided glob-style pattern. Return
True
if matching is successful,False
otherwise. For example:>>>PurePath('a/b.py').full_match('a/*.py')True>>>PurePath('a/b.py').full_match('*.py')False>>>PurePath('/a/b/c.py').full_match('/a/**')True>>>PurePath('/a/b/c.py').full_match('**/*.py')True
See also
Pattern language documentation.
As with other methods, case-sensitivity follows platform defaults:
>>>PurePosixPath('b.py').full_match('*.PY')False>>>PureWindowsPath('b.py').full_match('*.PY')True
Setcase_sensitive to
True
orFalse
to override this behaviour.Added in version 3.13.
- PurePath.match(pattern,*,case_sensitive=None)¶
Match this path against the provided non-recursive glob-style pattern.Return
True
if matching is successful,False
otherwise.This method is similar to
full_match()
, but empty patternsaren’t allowed (ValueError
is raised), the recursive wildcard“**
” isn’t supported (it acts like non-recursive “*
”), and if arelative pattern is provided, then matching is done from the right:>>>PurePath('a/b.py').match('*.py')True>>>PurePath('/a/b/c.py').match('b/*.py')True>>>PurePath('/a/b/c.py').match('a/*.py')False
Changed in version 3.12:Thepattern parameter accepts apath-like object.
Changed in version 3.12:Thecase_sensitive parameter was added.
- PurePath.relative_to(other,walk_up=False)¶
Compute a version of this path relative to the path represented byother. If it’s impossible,
ValueError
is raised:>>>p=PurePosixPath('/etc/passwd')>>>p.relative_to('/')PurePosixPath('etc/passwd')>>>p.relative_to('/etc')PurePosixPath('passwd')>>>p.relative_to('/usr')Traceback (most recent call last): File"<stdin>", line1, in<module> File"pathlib.py", line941, inrelative_toraiseValueError(error_message.format(str(self),str(formatted)))ValueError:'/etc/passwd' is not in the subpath of '/usr' OR one path is relative and the other is absolute.
Whenwalk_up is false (the default), the path must start withother.When the argument is true,
..
entries may be added to form therelative path. In all other cases, such as the paths referencingdifferent drives,ValueError
is raised.:>>>p.relative_to('/usr',walk_up=True)PurePosixPath('../etc/passwd')>>>p.relative_to('foo',walk_up=True)Traceback (most recent call last): File"<stdin>", line1, in<module> File"pathlib.py", line941, inrelative_toraiseValueError(error_message.format(str(self),str(formatted)))ValueError:'/etc/passwd' is not on the same drive as 'foo' OR one path is relative and the other is absolute.
Warning
This function is part of
PurePath
and works with strings.It does not check or access the underlying file structure.This can impact thewalk_up option as it assumes that no symlinksare present in the path; callresolve()
first ifnecessary to resolve symlinks.Changed in version 3.12:Thewalk_up parameter was added (old behavior is the same as
walk_up=False
).Deprecated since version 3.12, will be removed in version 3.14:Passing additional positional arguments is deprecated; if supplied,they are joined withother.
- PurePath.with_name(name)¶
Return a new path with the
name
changed. If the original pathdoesn’t have a name, ValueError is raised:>>>p=PureWindowsPath('c:/Downloads/pathlib.tar.gz')>>>p.with_name('setup.py')PureWindowsPath('c:/Downloads/setup.py')>>>p=PureWindowsPath('c:/')>>>p.with_name('setup.py')Traceback (most recent call last): File"<stdin>", line1, in<module> File"/home/antoine/cpython/default/Lib/pathlib.py", line751, inwith_nameraiseValueError("%r has an empty name"%(self,))ValueError:PureWindowsPath('c:/') has an empty name
- PurePath.with_stem(stem)¶
Return a new path with the
stem
changed. If the original pathdoesn’t have a name, ValueError is raised:>>>p=PureWindowsPath('c:/Downloads/draft.txt')>>>p.with_stem('final')PureWindowsPath('c:/Downloads/final.txt')>>>p=PureWindowsPath('c:/Downloads/pathlib.tar.gz')>>>p.with_stem('lib')PureWindowsPath('c:/Downloads/lib.gz')>>>p=PureWindowsPath('c:/')>>>p.with_stem('')Traceback (most recent call last): File"<stdin>", line1, in<module> File"/home/antoine/cpython/default/Lib/pathlib.py", line861, inwith_stemreturnself.with_name(stem+self.suffix) File"/home/antoine/cpython/default/Lib/pathlib.py", line851, inwith_nameraiseValueError("%r has an empty name"%(self,))ValueError:PureWindowsPath('c:/') has an empty name
Added in version 3.9.
- PurePath.with_suffix(suffix)¶
Return a new path with the
suffix
changed. If the original pathdoesn’t have a suffix, the newsuffix is appended instead. If thesuffix is an empty string, the original suffix is removed:>>>p=PureWindowsPath('c:/Downloads/pathlib.tar.gz')>>>p.with_suffix('.bz2')PureWindowsPath('c:/Downloads/pathlib.tar.bz2')>>>p=PureWindowsPath('README')>>>p.with_suffix('.txt')PureWindowsPath('README.txt')>>>p=PureWindowsPath('README.txt')>>>p.with_suffix('')PureWindowsPath('README')
- PurePath.with_segments(*pathsegments)¶
Create a new path object of the same type by combining the givenpathsegments. This method is called whenever a derivative path is created,such as from
parent
andrelative_to()
. Subclasses mayoverride this method to pass information to derivative paths, for example:frompathlibimportPurePosixPathclassMyPath(PurePosixPath):def__init__(self,*pathsegments,session_id):super().__init__(*pathsegments)self.session_id=session_iddefwith_segments(self,*pathsegments):returntype(self)(*pathsegments,session_id=self.session_id)etc=MyPath('/etc',session_id=42)hosts=etc/'hosts'print(hosts.session_id)# 42
Added in version 3.12.
Concrete paths¶
Concrete paths are subclasses of the pure path classes. In addition tooperations provided by the latter, they also provide methods to do systemcalls on path objects. There are three ways to instantiate concrete paths:
- classpathlib.Path(*pathsegments)¶
A subclass of
PurePath
, this class represents concrete paths ofthe system’s path flavour (instantiating it creates either aPosixPath
or aWindowsPath
):>>>Path('setup.py')PosixPath('setup.py')
pathsegments is specified similarly to
PurePath
.
- classpathlib.PosixPath(*pathsegments)¶
A subclass of
Path
andPurePosixPath
, this classrepresents concrete non-Windows filesystem paths:>>>PosixPath('/etc/hosts')PosixPath('/etc/hosts')
pathsegments is specified similarly to
PurePath
.Changed in version 3.13:Raises
UnsupportedOperation
on Windows. In previous versions,NotImplementedError
was raised instead.
- classpathlib.WindowsPath(*pathsegments)¶
A subclass of
Path
andPureWindowsPath
, this classrepresents concrete Windows filesystem paths:>>>WindowsPath('c:/','Users','Ximénez')WindowsPath('c:/Users/Ximénez')
pathsegments is specified similarly to
PurePath
.Changed in version 3.13:Raises
UnsupportedOperation
on non-Windows platforms. In previousversions,NotImplementedError
was raised instead.
You can only instantiate the class flavour that corresponds to your system(allowing system calls on non-compatible path flavours could lead tobugs or failures in your application):
>>>importos>>>os.name'posix'>>>Path('setup.py')PosixPath('setup.py')>>>PosixPath('setup.py')PosixPath('setup.py')>>>WindowsPath('setup.py')Traceback (most recent call last): File"<stdin>", line1, in<module> File"pathlib.py", line798, in__new__%(cls.__name__,))UnsupportedOperation:cannot instantiate 'WindowsPath' on your system
Some concrete path methods can raise anOSError
if a system call fails(for example because the path doesn’t exist).
Parsing and generating URIs¶
Concrete path objects can be created from, and represented as, ‘file’ URIsconforming toRFC 8089.
Note
File URIs are not portable across machines with differentfilesystem encodings.
- classmethodPath.from_uri(uri)¶
Return a new path object from parsing a ‘file’ URI. For example:
>>>p=Path.from_uri('file:///etc/hosts')PosixPath('/etc/hosts')
On Windows, DOS device and UNC paths may be parsed from URIs:
>>>p=Path.from_uri('file:///c:/windows')WindowsPath('c:/windows')>>>p=Path.from_uri('file://server/share')WindowsPath('//server/share')
Several variant forms are supported:
>>>p=Path.from_uri('file:////server/share')WindowsPath('//server/share')>>>p=Path.from_uri('file://///server/share')WindowsPath('//server/share')>>>p=Path.from_uri('file:c:/windows')WindowsPath('c:/windows')>>>p=Path.from_uri('file:/c|/windows')WindowsPath('c:/windows')
ValueError
is raised if the URI does not start withfile:
, orthe parsed path isn’t absolute.Added in version 3.13.
- Path.as_uri()¶
Represent the path as a ‘file’ URI.
ValueError
is raised ifthe path isn’t absolute.>>>p=PosixPath('/etc/passwd')>>>p.as_uri()'file:///etc/passwd'>>>p=WindowsPath('c:/Windows')>>>p.as_uri()'file:///c:/Windows'
For historical reasons, this method is also available from
PurePath
objects. However, its use ofos.fsencode()
makesit strictly impure.
Expanding and resolving paths¶
- classmethodPath.home()¶
Return a new path object representing the user’s home directory (asreturned by
os.path.expanduser()
with~
construct). If the homedirectory can’t be resolved,RuntimeError
is raised.>>>Path.home()PosixPath('/home/antoine')
Added in version 3.5.
- Path.expanduser()¶
Return a new path with expanded
~
and~user
constructs,as returned byos.path.expanduser()
. If a home directory can’t beresolved,RuntimeError
is raised.>>>p=PosixPath('~/films/Monty Python')>>>p.expanduser()PosixPath('/home/eric/films/Monty Python')
Added in version 3.5.
- classmethodPath.cwd()¶
Return a new path object representing the current directory (as returnedby
os.getcwd()
):>>>Path.cwd()PosixPath('/home/antoine/pathlib')
- Path.absolute()¶
Make the path absolute, without normalization or resolving symlinks.Returns a new path object:
>>>p=Path('tests')>>>pPosixPath('tests')>>>p.absolute()PosixPath('/home/antoine/pathlib/tests')
- Path.resolve(strict=False)¶
Make the path absolute, resolving any symlinks. A new path object isreturned:
>>>p=Path()>>>pPosixPath('.')>>>p.resolve()PosixPath('/home/antoine/pathlib')
“
..
” components are also eliminated (this is the only method to do so):>>>p=Path('docs/../setup.py')>>>p.resolve()PosixPath('/home/antoine/pathlib/setup.py')
If a path doesn’t exist or a symlink loop is encountered, andstrict is
True
,OSError
is raised. Ifstrict isFalse
, the path isresolved as far as possible and any remainder is appended without checkingwhether it exists.Changed in version 3.6:Thestrict parameter was added (pre-3.6 behavior is strict).
Changed in version 3.13:Symlink loops are treated like other errors:
OSError
is raised instrict mode, and no exception is raised in non-strict mode. In previousversions,RuntimeError
is raised no matter the value ofstrict.
- Path.readlink()¶
Return the path to which the symbolic link points (as returned by
os.readlink()
):>>>p=Path('mylink')>>>p.symlink_to('setup.py')>>>p.readlink()PosixPath('setup.py')
Added in version 3.9.
Changed in version 3.13:Raises
UnsupportedOperation
ifos.readlink()
is notavailable. In previous versions,NotImplementedError
was raised.
Querying file type and status¶
Changed in version 3.8:exists()
,is_dir()
,is_file()
,is_mount()
,is_symlink()
,is_block_device()
,is_char_device()
,is_fifo()
,is_socket()
now returnFalse
instead of raising an exception for paths that contain charactersunrepresentable at the OS level.
- Path.stat(*,follow_symlinks=True)¶
Return an
os.stat_result
object containing information about this path, likeos.stat()
.The result is looked up at each call to this method.This method normally follows symlinks; to stat a symlink add the argument
follow_symlinks=False
, or uselstat()
.>>>p=Path('setup.py')>>>p.stat().st_size956>>>p.stat().st_mtime1327883547.852554
Changed in version 3.10:Thefollow_symlinks parameter was added.
- Path.lstat()¶
Like
Path.stat()
but, if the path points to a symbolic link, returnthe symbolic link’s information rather than its target’s.
- Path.exists(*,follow_symlinks=True)¶
Return
True
if the path points to an existing file or directory.This method normally follows symlinks; to check if a symlink exists, addthe argument
follow_symlinks=False
.>>>Path('.').exists()True>>>Path('setup.py').exists()True>>>Path('/etc').exists()True>>>Path('nonexistentfile').exists()False
Changed in version 3.12:Thefollow_symlinks parameter was added.
- Path.is_file(*,follow_symlinks=True)¶
Return
True
if the path points to a regular file,False
if itpoints to another kind of file.False
is also returned if the path doesn’t exist or is a broken symlink;other errors (such as permission errors) are propagated.This method normally follows symlinks; to exclude symlinks, add theargument
follow_symlinks=False
.Changed in version 3.13:Thefollow_symlinks parameter was added.
- Path.is_dir(*,follow_symlinks=True)¶
Return
True
if the path points to a directory,False
if it pointsto another kind of file.False
is also returned if the path doesn’t exist or is a broken symlink;other errors (such as permission errors) are propagated.This method normally follows symlinks; to exclude symlinks to directories,add the argument
follow_symlinks=False
.Changed in version 3.13:Thefollow_symlinks parameter was added.
- Path.is_symlink()¶
Return
True
if the path points to a symbolic link,False
otherwise.False
is also returned if the path doesn’t exist; other errors (suchas permission errors) are propagated.
- Path.is_junction()¶
Return
True
if the path points to a junction, andFalse
for any othertype of file. Currently only Windows supports junctions.Added in version 3.12.
- Path.is_mount()¶
Return
True
if the path is amount point: a point in afile system where a different file system has been mounted. On POSIX, thefunction checks whetherpath’s parent,path/..
, is on a differentdevice thanpath, or whetherpath/..
andpath point to the samei-node on the same device — this should detect mount points for all Unixand POSIX variants. On Windows, a mount point is considered to be a driveletter root (e.g.c:\
), a UNC share (e.g.\\server\share
), or amounted filesystem directory.Added in version 3.7.
Changed in version 3.12:Windows support was added.
- Path.is_socket()¶
Return
True
if the path points to a Unix socket (or a symbolic linkpointing to a Unix socket),False
if it points to another kind of file.False
is also returned if the path doesn’t exist or is a broken symlink;other errors (such as permission errors) are propagated.
- Path.is_fifo()¶
Return
True
if the path points to a FIFO (or a symbolic linkpointing to a FIFO),False
if it points to another kind of file.False
is also returned if the path doesn’t exist or is a broken symlink;other errors (such as permission errors) are propagated.
- Path.is_block_device()¶
Return
True
if the path points to a block device (or a symbolic linkpointing to a block device),False
if it points to another kind of file.False
is also returned if the path doesn’t exist or is a broken symlink;other errors (such as permission errors) are propagated.
- Path.is_char_device()¶
Return
True
if the path points to a character device (or a symbolic linkpointing to a character device),False
if it points to another kind of file.False
is also returned if the path doesn’t exist or is a broken symlink;other errors (such as permission errors) are propagated.
- Path.samefile(other_path)¶
Return whether this path points to the same file asother_path, whichcan be either a Path object, or a string. The semantics are similarto
os.path.samefile()
andos.path.samestat()
.An
OSError
can be raised if either file cannot be accessed for somereason.>>>p=Path('spam')>>>q=Path('eggs')>>>p.samefile(q)False>>>p.samefile('spam')True
Added in version 3.5.
Reading and writing files¶
- Path.open(mode='r',buffering=-1,encoding=None,errors=None,newline=None)¶
Open the file pointed to by the path, like the built-in
open()
function does:>>>p=Path('setup.py')>>>withp.open()asf:...f.readline()...'#!/usr/bin/env python3\n'
- Path.read_text(encoding=None,errors=None,newline=None)¶
Return the decoded contents of the pointed-to file as a string:
>>>p=Path('my_text_file')>>>p.write_text('Text file contents')18>>>p.read_text()'Text file contents'
The file is opened and then closed. The optional parameters have the samemeaning as in
open()
.Added in version 3.5.
Changed in version 3.13:Thenewline parameter was added.
- Path.read_bytes()¶
Return the binary contents of the pointed-to file as a bytes object:
>>>p=Path('my_binary_file')>>>p.write_bytes(b'Binary file contents')20>>>p.read_bytes()b'Binary file contents'
Added in version 3.5.
- Path.write_text(data,encoding=None,errors=None,newline=None)¶
Open the file pointed to in text mode, writedata to it, and close thefile:
>>>p=Path('my_text_file')>>>p.write_text('Text file contents')18>>>p.read_text()'Text file contents'
An existing file of the same name is overwritten. The optional parametershave the same meaning as in
open()
.Added in version 3.5.
Changed in version 3.10:Thenewline parameter was added.
- Path.write_bytes(data)¶
Open the file pointed to in bytes mode, writedata to it, and close thefile:
>>>p=Path('my_binary_file')>>>p.write_bytes(b'Binary file contents')20>>>p.read_bytes()b'Binary file contents'
An existing file of the same name is overwritten.
Added in version 3.5.
Reading directories¶
- Path.iterdir()¶
When the path points to a directory, yield path objects of the directorycontents:
>>>p=Path('docs')>>>forchildinp.iterdir():child...PosixPath('docs/conf.py')PosixPath('docs/_templates')PosixPath('docs/make.bat')PosixPath('docs/index.rst')PosixPath('docs/_build')PosixPath('docs/_static')PosixPath('docs/Makefile')
The children are yielded in arbitrary order, and the special entries
'.'
and'..'
are not included. If a file is removed from or addedto the directory after creating the iterator, it is unspecified whethera path object for that file is included.If the path is not a directory or otherwise inaccessible,
OSError
israised.
- Path.glob(pattern,*,case_sensitive=None,recurse_symlinks=False)¶
Glob the given relativepattern in the directory represented by this path,yielding all matching files (of any kind):
>>>sorted(Path('.').glob('*.py'))[PosixPath('pathlib.py'), PosixPath('setup.py'), PosixPath('test_pathlib.py')]>>>sorted(Path('.').glob('*/*.py'))[PosixPath('docs/conf.py')]>>>sorted(Path('.').glob('**/*.py'))[PosixPath('build/lib/pathlib.py'), PosixPath('docs/conf.py'), PosixPath('pathlib.py'), PosixPath('setup.py'), PosixPath('test_pathlib.py')]
See also
Pattern language documentation.
By default, or when thecase_sensitive keyword-only argument is set to
None
, this method matches paths using platform-specific casing rules:typically, case-sensitive on POSIX, and case-insensitive on Windows.Setcase_sensitive toTrue
orFalse
to override this behaviour.By default, or when therecurse_symlinks keyword-only argument is set to
False
, this method follows symlinks except when expanding “**
”wildcards. Setrecurse_symlinks toTrue
to always follow symlinks.Raises anauditing event
pathlib.Path.glob
with argumentsself
,pattern
.Changed in version 3.12:Thecase_sensitive parameter was added.
Changed in version 3.13:Therecurse_symlinks parameter was added.
Changed in version 3.13:Thepattern parameter accepts apath-like object.
Changed in version 3.13:Any
OSError
exceptions raised from scanning the filesystem aresuppressed. In previous versions, such exceptions are suppressed in manycases, but not all.
- Path.rglob(pattern,*,case_sensitive=None,recurse_symlinks=False)¶
Glob the given relativepattern recursively. This is like calling
Path.glob()
with “**/
” added in front of thepattern.See also
Pattern language and
Path.glob()
documentation.Raises anauditing event
pathlib.Path.rglob
with argumentsself
,pattern
.Changed in version 3.12:Thecase_sensitive parameter was added.
Changed in version 3.13:Therecurse_symlinks parameter was added.
Changed in version 3.13:Thepattern parameter accepts apath-like object.
- Path.walk(top_down=True,on_error=None,follow_symlinks=False)¶
Generate the file names in a directory tree by walking the treeeither top-down or bottom-up.
For each directory in the directory tree rooted atself (includingself but excluding ‘.’ and ‘..’), the method yields a 3-tuple of
(dirpath,dirnames,filenames)
.dirpath is a
Path
to the directory currently being walked,dirnames is a list of strings for the names of subdirectories indirpath(excluding'.'
and'..'
), andfilenames is a list of strings forthe names of the non-directory files indirpath. To get a full path(which begins withself) to a file or directory indirpath, dodirpath/name
. Whether or not the lists are sorted is filesystem-dependent.If the optional argumenttop_down is true (which is the default), the triple for adirectory is generated before the triples for any of its subdirectories(directories are walked top-down). Iftop_down is false, the triplefor a directory is generated after the triples for all of its subdirectories(directories are walked bottom-up). No matter the value oftop_down, thelist of subdirectories is retrieved before the triples for the directory andits subdirectories are walked.
Whentop_down is true, the caller can modify thedirnames list in-place(for example, using
del
or slice assignment), andPath.walk()
will only recurse into the subdirectories whose names remain indirnames.This can be used to prune the search, or to impose a specific order of visiting,or even to informPath.walk()
about directories the caller creates orrenames before it resumesPath.walk()
again. Modifyingdirnames whentop_down is false has no effect on the behavior ofPath.walk()
since thedirectories indirnames have already been generated by the timedirnamesis yielded to the caller.By default, errors from
os.scandir()
are ignored. If the optionalargumenton_error is specified, it should be a callable; it will becalled with one argument, anOSError
instance. The callable can handle theerror to continue the walk or re-raise it to stop the walk. Note that thefilename is available as thefilename
attribute of the exception object.By default,
Path.walk()
does not follow symbolic links, and instead adds themto thefilenames list. Setfollow_symlinks to true to resolve symlinksand place them indirnames andfilenames as appropriate for their targets, andconsequently visit directories pointed to by symlinks (where supported).Note
Be aware that settingfollow_symlinks to true can lead to infiniterecursion if a link points to a parent directory of itself.
Path.walk()
does not keep track of the directories it has already visited.Note
Path.walk()
assumes the directories it walks are not modified duringexecution. For example, if a directory fromdirnames has been replacedwith a symlink andfollow_symlinks is false,Path.walk()
willstill try to descend into it. To prevent such behavior, remove directoriesfromdirnames as appropriate.Note
Unlike
os.walk()
,Path.walk()
lists symlinks to directories infilenames iffollow_symlinks is false.This example displays the number of bytes used by all files in each directory,while ignoring
__pycache__
directories:frompathlibimportPathforroot,dirs,filesinPath("cpython/Lib/concurrent").walk(on_error=print):print(root,"consumes",sum((root/file).stat().st_sizeforfileinfiles),"bytes in",len(files),"non-directory files")if'__pycache__'indirs:dirs.remove('__pycache__')
This next example is a simple implementation of
shutil.rmtree()
.Walking the tree bottom-up is essential asrmdir()
doesn’t allowdeleting a directory before it is empty:# Delete everything reachable from the directory "top".# CAUTION: This is dangerous! For example, if top == Path('/'),# it could delete all of your files.forroot,dirs,filesintop.walk(top_down=False):fornameinfiles:(root/name).unlink()fornameindirs:(root/name).rmdir()
Added in version 3.12.
Creating files and directories¶
- Path.touch(mode=0o666,exist_ok=True)¶
Create a file at this given path. Ifmode is given, it is combinedwith the process’s
umask
value to determine the file mode and accessflags. If the file already exists, the function succeeds whenexist_okis true (and its modification time is updated to the current time),otherwiseFileExistsError
is raised.See also
The
open()
,write_text()
andwrite_bytes()
methods are often used to create files.
- Path.mkdir(mode=0o777,parents=False,exist_ok=False)¶
Create a new directory at this given path. Ifmode is given, it iscombined with the process’s
umask
value to determine the file modeand access flags. If the path already exists,FileExistsError
is raised.Ifparents is true, any missing parents of this path are createdas needed; they are created with the default permissions without takingmode into account (mimicking the POSIX
mkdir-p
command).Ifparents is false (the default), a missing parent raises
FileNotFoundError
.Ifexist_ok is false (the default),
FileExistsError
israised if the target directory already exists.Ifexist_ok is true,
FileExistsError
will not be raised unless the givenpath already exists in the file system and is not a directory (samebehavior as the POSIXmkdir-p
command).Changed in version 3.5:Theexist_ok parameter was added.
- Path.symlink_to(target,target_is_directory=False)¶
Make this path a symbolic link pointing totarget.
On Windows, a symlink represents either a file or a directory, and does notmorph to the target dynamically. If the target is present, the type of thesymlink will be created to match. Otherwise, the symlink will be createdas a directory iftarget_is_directory is true or a file symlink (thedefault) otherwise. On non-Windows platforms,target_is_directory is ignored.
>>>p=Path('mylink')>>>p.symlink_to('setup.py')>>>p.resolve()PosixPath('/home/antoine/pathlib/setup.py')>>>p.stat().st_size956>>>p.lstat().st_size8
Note
The order of arguments (link, target) is the reverseof
os.symlink()
’s.Changed in version 3.13:Raises
UnsupportedOperation
ifos.symlink()
is notavailable. In previous versions,NotImplementedError
was raised.
- Path.hardlink_to(target)¶
Make this path a hard link to the same file astarget.
Note
The order of arguments (link, target) is the reverseof
os.link()
’s.Added in version 3.10.
Changed in version 3.13:Raises
UnsupportedOperation
ifos.link()
is notavailable. In previous versions,NotImplementedError
was raised.
Renaming and deleting¶
- Path.rename(target)¶
Rename this file or directory to the giventarget, and return a new
Path
instance pointing totarget. On Unix, iftarget existsand is a file, it will be replaced silently if the user has permission.On Windows, iftarget exists,FileExistsError
will be raised.target can be either a string or another path object:>>>p=Path('foo')>>>p.open('w').write('some text')9>>>target=Path('bar')>>>p.rename(target)PosixPath('bar')>>>target.open().read()'some text'
The target path may be absolute or relative. Relative paths are interpretedrelative to the current working directory,not the directory of the
Path
object.It is implemented in terms of
os.rename()
and gives the same guarantees.Changed in version 3.8:Added return value, return the new
Path
instance.
- Path.replace(target)¶
Rename this file or directory to the giventarget, and return a new
Path
instance pointing totarget. Iftarget points to anexisting file or empty directory, it will be unconditionally replaced.The target path may be absolute or relative. Relative paths are interpretedrelative to the current working directory,not the directory of the
Path
object.Changed in version 3.8:Added return value, return the new
Path
instance.
- Path.unlink(missing_ok=False)¶
Remove this file or symbolic link. If the path points to a directory,use
Path.rmdir()
instead.Ifmissing_ok is false (the default),
FileNotFoundError
israised if the path does not exist.Ifmissing_ok is true,
FileNotFoundError
exceptions will beignored (same behavior as the POSIXrm-f
command).Changed in version 3.8:Themissing_ok parameter was added.
- Path.rmdir()¶
Remove this directory. The directory must be empty.
Permissions and ownership¶
- Path.owner(*,follow_symlinks=True)¶
Return the name of the user owning the file.
KeyError
is raisedif the file’s user identifier (UID) isn’t found in the system database.This method normally follows symlinks; to get the owner of the symlink, addthe argument
follow_symlinks=False
.Changed in version 3.13:Raises
UnsupportedOperation
if thepwd
module is notavailable. In earlier versions,NotImplementedError
was raised.Changed in version 3.13:Thefollow_symlinks parameter was added.
- Path.group(*,follow_symlinks=True)¶
Return the name of the group owning the file.
KeyError
is raisedif the file’s group identifier (GID) isn’t found in the system database.This method normally follows symlinks; to get the group of the symlink, addthe argument
follow_symlinks=False
.Changed in version 3.13:Raises
UnsupportedOperation
if thegrp
module is notavailable. In earlier versions,NotImplementedError
was raised.Changed in version 3.13:Thefollow_symlinks parameter was added.
- Path.chmod(mode,*,follow_symlinks=True)¶
Change the file mode and permissions, like
os.chmod()
.This method normally follows symlinks. Some Unix flavours support changingpermissions on the symlink itself; on these platforms you may add theargument
follow_symlinks=False
, or uselchmod()
.>>>p=Path('setup.py')>>>p.stat().st_mode33277>>>p.chmod(0o444)>>>p.stat().st_mode33060
Changed in version 3.10:Thefollow_symlinks parameter was added.
- Path.lchmod(mode)¶
Like
Path.chmod()
but, if the path points to a symbolic link, thesymbolic link’s mode is changed rather than its target’s.
Pattern language¶
The following wildcards are supported in patterns forfull_match()
,glob()
andrglob()
:
**
(entire segment)Matches any number of file or directory segments, including zero.
*
(entire segment)Matches one file or directory segment.
*
(part of a segment)Matches any number of non-separator characters, including zero.
?
Matches one non-separator character.
[seq]
Matches one character inseq, whereseq is a sequence of characters.Range expressions are supported; for example,
[a-z]
matches any lowercase ASCII letter.Multiple ranges can be combined:[a-zA-Z0-9_]
matches any ASCII letter, digit, or underscore.[!seq]
Matches one character not inseq, whereseq follows the same rules as above.
For a literal match, wrap the meta-characters in brackets.For example,"[?]"
matches the character"?"
.
The “**
” wildcard enables recursive globbing. A few examples:
Pattern | Meaning |
---|---|
“ | Any path with at least one segment. |
“ | Any path with a final segment ending “ |
“ | Any path starting with “ |
“ | Any path starting with “ |
Note
Globbing with the “**
” wildcard visits every directory in the tree.Large directory trees may take a long time to search.
Changed in version 3.13:Globbing with a pattern that ends with “**
” returns both files anddirectories. In previous versions, only directories were returned.
InPath.glob()
andrglob()
, a trailing slash may be added tothe pattern to match only directories.
Comparison to theglob
module¶
The patterns accepted and results generated byPath.glob()
andPath.rglob()
differ slightly from those by theglob
module:
Files beginning with a dot are not special in pathlib. This islike passing
include_hidden=True
toglob.glob()
.“
**
” pattern components are always recursive in pathlib. This is likepassingrecursive=True
toglob.glob()
.“
**
” pattern components do not follow symlinks by default in pathlib.This behaviour has no equivalent inglob.glob()
, but you can passrecurse_symlinks=True
toPath.glob()
for compatible behaviour.Like all
PurePath
andPath
objects, the values returnedfromPath.glob()
andPath.rglob()
don’t include trailingslashes.The values returned from pathlib’s
path.glob()
andpath.rglob()
include thepath as a prefix, unlike the results ofglob.glob(root_dir=path)
.The values returned from pathlib’s
path.glob()
andpath.rglob()
may includepath itself, for example when globbing “**
”, whereas theresults ofglob.glob(root_dir=path)
never include an empty string thatwould correspond topath.
Comparison to theos
andos.path
modules¶
pathlib implements path operations usingPurePath
andPath
objects, and so it’s said to beobject-oriented. On the other hand, theos
andos.path
modules supply functions that work with low-levelstr
andbytes
objects, which is a moreprocedural approach. Someusers consider the object-oriented style to be more readable.
Many functions inos
andos.path
supportbytes
paths andpaths relative to directory descriptors. These features aren’tavailable in pathlib.
Python’sstr
andbytes
types, and portions of theos
andos.path
modules, are written in C and are very speedy. pathlib iswritten in pure Python and is often slower, but rarely slow enough to matter.
pathlib’s path normalization is slightly more opinionated and consistent thanos.path
. For example, whereasos.path.abspath()
eliminates“..
” segments from a path, which may change its meaning if symlinks areinvolved,Path.absolute()
preserves these segments for greater safety.
pathlib’s path normalization may render it unsuitable for some applications:
pathlib normalizes
Path("my_folder/")
toPath("my_folder")
, whichchanges a path’s meaning when supplied to various operating system APIs andcommand-line utilities. Specifically, the absence of a trailing separatormay allow the path to be resolved as either a file or directory, ratherthan a directory only.pathlib normalizes
Path("./my_program")
toPath("my_program")
,which changes a path’s meaning when used as an executable search path, suchas in a shell or when spawning a child process. Specifically, the absenceof a separator in the path may force it to be looked up inPATH
rather than the current directory.
As a consequence of these differences, pathlib is not a drop-in replacementforos.path
.
Corresponding tools¶
Below is a table mapping variousos
functions to their correspondingPurePath
/Path
equivalent.
Footnotes
[1]os.path.relpath()
callsabspath()
to make pathsabsolute and remove “..
” parts, whereasPurePath.relative_to()
is a lexical operation that raisesValueError
when its inputs’anchors differ (e.g. if one path is absolute and the other relative.)
os.path.expanduser()
returns the path unchanged if the homedirectory can’t be resolved, whereasPath.expanduser()
raisesRuntimeError
.
os.path.abspath()
removes “..
” components without resolvingsymlinks, which may change the meaning of the path, whereasPath.absolute()
leaves any “..
” components in the path.
os.walk()
always follows symlinks when categorizing paths intodirnames andfilenames, whereasPath.walk()
categorizes allsymlinks intofilenames whenfollow_symlinks is false (the default.)