- Notifications
You must be signed in to change notification settings - Fork0
Zircon is an extensible and user-friendly, multiplatform tile engine.
License
gustafssonlinnea/zircon
Folders and files
Name | Name | Last commit message | Last commit date | |
---|---|---|---|---|
Repository files navigation
Need info? Check theDocs| orCreate an issue| Checkour project Board|Ask us on Discord| Support us onPatreon|Javadoc / Kdoc
If you want to start working with Zircon you can either add it to your project as a Maven dependency or you can try outthe skeleton projects(Java,Kotlin)which come with batteries included.
The officialdocumentation site contains a lot of information. The examples are also documented on theZircon Examples page(under construction), and the best place to start is theZircon Crash Course.
If you like learning by doing check out the source ofZircon fromhere and youcan run the examples for yourself. If you are usingJavastarthere. Alternatively if you useKotlin the code can befoundhere.
If you just want to peruse theZircon API navigatehere. Everything which is intended to be part of the publicAPI is there.
You can find theJavadoc /Kdochere
If you'd like to talk to us, join us on ourDiscord Server.
Maven:
<dependencies> <dependency> <groupId>org.hexworks.zircon</groupId> <artifactId>zircon.core-jvm</artifactId> <version>2021.1.0-RELEASE</version> </dependency><!-- use zircon.jvm.libgdx if you want to use LibGDX instead of Swing--> <dependency> <groupId>org.hexworks.zircon</groupId> <artifactId>zircon.jvm.swing</artifactId> <version>2021.1.0-RELEASE</version> </dependency></dependencies>
Gradle:
dependencies { implementation"org.hexworks.zircon:zircon.core-jvm:2021.1.0-RELEASE" implementation"org.hexworks.zircon:zircon.jvm.swing:2021.1.0-RELEASE"}
Once you have the dependencies set up you can start usingZircon by creating aTileGrid
:
publicclassMain {publicstaticvoidmain(String[]args) {// a TileGrid represents a 2D grid composed of TilesTileGridtileGrid =SwingApplications.startTileGrid(AppConfig.newBuilder()// The number of tiles horizontally, and vertically .withSize(60,30)// You can choose from a wide array of CP437, True Type or Graphical tilesets// that are built into Zircon .withDefaultTileset(CP437TilesetResources.rexPaint16x16()) .build());// A Screen is an abstraction that lets you use text GUI Components// You can have multiple Screens attached to the same TileGrid to be able to create multiple// screens for your app.Screenscreen =Screen.create(tileGrid);// Creating text GUI Components is super simpleLabellabel =Components.label() .withText("Hello, Zircon!") .withAlignment(ComponentAlignments.alignmentWithin(tileGrid,ComponentAlignment.CENTER)) .build();// Screens can hold GUI componentsscreen.addComponent(label);// Displaying a screen will make it visible. It will also hide a previously shown Screen.screen.display();// Zircon comes with a plethora of built-in color themesscreen.setTheme(ColorThemes.arc()); }}
The output of this example is:
Congratulations! Now you are aZircon user.
The following are some guidelines that can help you if you getstuck:
If you want to build something (aTileGraphics
, aComponent
or anything that is part of the public API) it isalmost sure that there is aBuilder
or a factory object for it. Each type that has a builder will have anewBuilder
function you can call to create the corresponding builder:Tile.newBuilder()
.
If there are multiple classes of objects that can be created there might also be a utility class,likeShapes
to create differentShape
objects. Your IDE will help you with this.
These classes reside in theorg.hexworks.zircon.api
package. There are some classes that are grouped together into asingle utility class, however. WithComponents
for example, you can obtainBuilder
s for allComponent
slikeComponents.panel()
orComponents.checkBox()
. Likewise, you can useDrawSurfaces
to obtain buildersforTileGraphics
andTileImage
.
If you want to work with external files like tilesets or REXPaint files check the same package(org.hexworks.zircon.api
), and look for classes that end with*Resources
. There are a bunch of built-in tilesetsfor example which you can choose from, but you can also load your own.
The rule of thumb is that if you need something external there is probably a*Resources
class for it (liketheCP437TilesetResources
).
You can useanything you can find in theAPI package, they are part of the public API, and safe to use.Theinternal package however is considered private toZircon so keep in mind that they can change anytime.
Some topics are explained in depth in thedocumentation.
If you want to see some example code take a look at the examples projecthere. Most examples haveidenticalJava andKotlin variants.
If all else fails read the javadocs. API classes are well documented.
If you have any problems that are not answered here feel free to ask us at theHexworks Discord server.
You can find detailed documentation about drawinghere.
The most basic operationZircon supports isdraw
ing. You can draw individualTile
s orTileGraphics
objects onyourTileGrid
. aTileGraphics
object is composed ofTile
s. This is a powerful tool, and you can implement morecomplex features using simpledraw
operations. In fact the component system is implemented on top of drawing, layeringand input handling features.
If you use REXPaint to design your programs, the good news is that you can import your.xp
files as well. Read moreabout ithere.
You can also useModifier
s in yourTile
s such asblink
,verticalFlip
orglow
. For a full list, checkthisfactory object.Modifier
s can either change the texture (like the ones above) or theTile
itself:
Read about input handling in the docshere.
Both theTileGrid
and theScreen
interfaces implementUIEventSource
which means that you can listen for userinputs using them. This includeskeystrokes andmouse input as well.
Both theTileGrid
and theScreen
interfaces implementLayerable
which means that you can addLayer
s on top ofthem. EveryLayerable
can have an arbitrary amount ofLayer
s.Layer
s are likeTileGraphics
objects, and you canalso have transparency in them which can be used to create fancy effects.Component
s are alsoLayer
s themselves. Take a look:
You can read more about the Component System on thedocumentation page.Color themes are detailedhere.
Component
s are GUI controls which can be used for showing content to the user (Label
s,Paragraph
s, etc.), enablingthem to interact with your program (Button
s,Slider
s, etc.) or to hold other components (Panel
s for example).
These components are rather simple, and you can expect them to work in a way you might be familiar with:
- You can click on them (press and release are different events).
- You can attach event listeners on them.
- Zircon implements focus handling, so you can navigate between the components using the
[Tab]
key(forwards) or the[Shift]+[Tab]
keystroke (backwards). - Components can be hovered, and you can also apply color themes to them.
What's more is that you can applyColorTheme
s toComponent
s. There are a bunch of built-in themes, and you can alsocreate your own.
To see a full list of availableComponent
s take a look at theComponentsfactory object or navigate to thecomponent docs page.
This is an example of how components look in action:
Read more about Animations in thedocs.
Animations are supported out of the box. You can either create them programmatically, or statically usingZircon's ownanimation format:.zap
(Zircon Animation Package). More about thathere. This is how an animationlooks like:
The shape documentation page can be foundhere.
You can drawShape
s like rectangles and triangles by using one of theShapeFactory
implementations. What's supportedout of the box istriangle,rectangle andline. The former two have filled versions as well. Check out theShapes
factory objecthere.
The documentation page for tilesets ishere.
Zircon comes with a bunch of built-in fonts tilesets. These come in 3 flavors:
Zircon also comes with its own tileset format (ztf
: Zircon Tileset Format) which isvery easy to use. It isdetailedhere.
If you want to see a new feature feel free tocreate a new Issue ordiscuss it with us onDiscord. Here are some features which are either under way or planned:
- Scrollable Components
- Menus
- Table Component
- Javadoc-style Documentation
- Grid / Screen Filters
- Floating Components
- Drag'n Drop Support
- Custom Layout Support
- Component Themes
- Custom Component Support
- Tree Component
- Accordion Component
- Combo Box Component
- IntelliJ Plugin
- Console for Zircon
If you'd like to give any of these a shot feel free tocontribute.
Zircon is made available under theApache 2.0 License.
Zircon is created and maintained by Addamsson, Coldwarrl, G3ldrin, Milonoir, Seveen and many others.
We're open to suggestions, feel free to message us onDiscord or open an issue.Pull requests are also welcome!
Zircon is powered by:
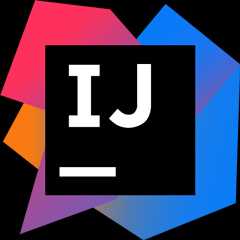
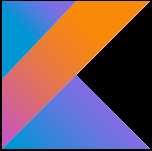
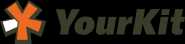
Thanks to the folks over atDwarf Fortress Tileset Repository forletting us bundle their tilesets.
Thanks toKyzrati who let us bundle theREXPaint Tilesets into Zircon!
Zircon comes bundled with theNethack Tileset.
Some True Type fonts are used fromGoogle Fonts.
Thanks toVileR for theOldschool Font Pack which we bundled into Zircon.
About
Zircon is an extensible and user-friendly, multiplatform tile engine.
Resources
License
Stars
Watchers
Forks
Packages0
Languages
- Kotlin93.7%
- Java6.3%